Reading and Writing State Variables in Solidity and Data Management in Smart Contracts
Contents
- 1 Reading and Writing State Variables in Solidity and Data Management in Smart Contracts
- 1.1 Chapter 1: Understanding State Variables in Solidity
- 1.1.1 Definition and Purpose of State Variables in Solidity
- 1.1.2 Purpose of State Variables:
- 1.1.3 Longevity and Immutability:
- 1.1.4 The Mechanics of State Variables:
- 1.1.5 Data Storage and Accessibility:
- 1.1.6 Declaration and Initialization:
- 1.1.7 Here is a typical example of declaring and initializing state variables in a Solidity contract:
- 1.1.8 Updating State Variables:
- 1.1.9 Conclusion of Section 1.2:
- 1.2 Chapter 2: Writing to State Variables
- 1.2.1 Transactional Dynamics of Writing to State Variables
- 1.2.2 Understanding Transactions:
- 1.2.3 Writing to State Variables:
- 1.2.4 Transaction Initiation:
- 1.2.5 Gas Estimation and Payment:
- 1.2.6 Example of a State-Modifying Function:
- 1.2.7 Challenges and Considerations:
- 1.2.8 Conclusion of Section:
- 1.2.9 Best Practices for Writing to State Variables in Solidity
- 1.2.10 1. Minimize State Changes:
- 1.2.11 2. Use Events for Non-Essential Data:
- 1.2.12 Example:
- 1.2.13 3. Optimize Function Modifiers and Conditions:
- 1.2.14 4. Batch Operations:
- 1.2.15 Example:
- 1.2.16 5. Gas Optimization Techniques:
- 1.2.17 Conclusion of Section 2.2:
- 1.3 Chapter 3: Reading from State Variables
- 1.3.1 Cost Efficiency of Reading Operations:
- 1.3.2 Zero Transaction Costs for Reads:
- 1.3.3 Using view and pure Functions:
- 1.3.4 Solidity provides two function types that are optimized for read operations:
- 1.3.5 Example of Efficient Read Operations:
- 1.3.6 Optimizing Client-Side Calls:
- 1.3.7 Strategic Use of Caching:
- 1.3.8 Conclusion of Section 3.1:
- 1.3.9 Strategic Implementation of Read Functions:
- 1.3.10 Optimizing Data Retrieval:
- 1.3.11 1. Designing Read Functions for Maximum Efficiency:
- 1.3.12 Example of an Efficient Read Function:
- 1.3.13 2. Leveraging view and pure Modifiers:
- 1.3.14 3. Caching Patterns for Frequent Reads:
- 1.3.15 4. Error Handling and Data Validation:
- 1.3.16 Conclusion of Section 3.2:
- 1.3.17 Conclusion:
- 1.3.18 Call to Action:
- 1.3.19 References
#EnterTheSmartContractSecuritySeries007
Abstract:
This academic exploration delves into the nuanced mechanisms of state variables within Solidity, the predominant programming language for Ethereum-based smart contracts. Focusing on the dual functionalities of reading from and writing to state variables, this article illuminates the foundational role these variables play in managing data across decentralized platforms. By detailing the processes and implications of data manipulation, this study aims to provide an in-depth understanding of the intrinsic behaviors of smart contracts, contributing significantly to the optimization of blockchain application development.
Introduction:
In the realm of blockchain technology, state variables represent a critical component in the architecture of smart contracts. Solidity, as the cornerstone language for Ethereum smart contract development, offers robust tools for state management that are essential for ensuring the consistency and reliability of decentralized applications (dApps). State variables in Solidity are not merely data holders; they are pivotal in maintaining the state and integrity of smart contracts across transactions. This paper examines the dynamics of reading from and writing to these variables, which is fundamental for developers aiming to engineer resilient and efficient dApps.
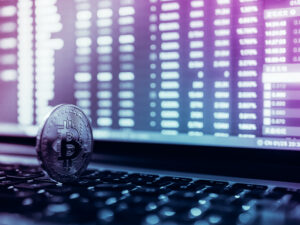
Reading and Writing State Variables in Solidity and Data Management in Smart Contracts
Chapter 1: Understanding State Variables in Solidity
Definition and Purpose of State Variables in Solidity
State variables are the primary means through which data permanence is achieved in smart contracts on the Ethereum blockchain. Defined within the contract and not within functions, these variables are stored directly on the blockchain, making their values persistent across all interactions and independent of the external environment. This permanence ensures that data remains consistent and reliable throughout the lifecycle of the contract, regardless of the state of the external world or the decentralized application it supports.
Purpose of State Variables:
The primary purpose of state variables in Solidity is to maintain the state of a smart contract. This state represents the collective current values of all data at any given time, encapsulating the history and present condition of the contract’s interactions. State variables facilitate several critical functions:
Data Storage: They store values such as user balances, application settings, and other necessary states required for the correct operation of decentralized applications.
Data Consistency: By retaining data across function calls and transactions, state variables ensure that the smart contract’s behavior is consistent and predictable. This consistency is crucial for trust and reliability in financial applications, voting systems, and other blockchain solutions that require a high degree of integrity.
Control Flow Management: State variables often control the logic flow within contracts by maintaining flags, counters, and other control elements that dictate the execution paths within the contract’s functions.
Longevity and Immutability:
Unlike local variables which reset after function execution, state variables remain until the smart contract is active on the blockchain. This permanence supports the design of complex decentralized systems that require historical data reference and long-term state management. Moreover, the immutability aspect of state variables (when declared as such) prevents tampering, providing an additional layer of security against contract manipulation.
In essence, state variables act as the memory bank of the smart contract, recording and storing all necessary information that defines the contract’s current state and governs its future behavior. This role is indispensable in the execution and management of reliable and effective smart contracts, underpinning the functionality of applications ranging from simple token storage mechanisms to intricate decentralized autonomous organizations (DAOs).
The Mechanics of State Variables:
/// SPDX-License-Identifier: MIT
pragma solidity ^0.8.17;
contract SimpleStorage {
uint public num; // Declare a state variable to store a number
// Function to update the state variable
function set(uint _num) public {
num = _num; // Writes to the state variable
}
// Function to read the state variable
function get() public view returns (uint) {
return num; // Reads the state variable without transaction costs
}
}
This code snippet demonstrates the SimpleStorage contract utilizing a state variable num. The set function updates num, while the get function reads num, illustrating the fundamental operations of state variables.1.2 The Mechanics of State Variables in Solidity
Data Storage and Accessibility:
State variables in Solidity are stored directly on the Ethereum blockchain, which not only provides durability and security but also ensures that these variables are accessible across all instances and executions of the smart contract. Unlike temporary variables that exist only during the execution of functions, state variables are permanently written to the blockchain’s data layer, making them a critical component of the contract’s architecture.
Declaration and Initialization:
State variables are declared at the top level of a contract and are often marked with visibility modifiers such as public, private, internal, or external to control access. The choice of modifier influences how these variables can be accessed and by whom, thus playing a key role in the contract’s security framework.
Here is a typical example of declaring and initializing state variables in a Solidity contract:
/// SPDX-License-Identifier: MIT
pragma solidity ^0.8.17;
contract AssetRegistry {
uint public assetCount; // Public state variable accessible by external entities
mapping(uint => address) private assetOwners; // Private state variable, mapping asset IDs to their owners
constructor() {
assetCount = 0; // Initialize the asset count when the contract is deployed
}
function registerAsset(address owner) public {
assetOwners[assetCount] = owner; // Assigns owner to the current asset count
assetCount++; // Increments the asset count
}
}
In this example, assetCount is a state variable used to keep track of the total number of assets registered in the contract, and it is publicly accessible. assetOwners is a mapping that stores the relationship between asset IDs and their respective owners, declared as private to restrict access to the contract itself.
Updating State Variables:
Updating state variables involves sending a transaction, which must be mined and included in the blockchain to take effect. This operation requires computational effort (gas) and forms the basis of Ethereum’s state transition function. Here’s how a state variable is typically updated:
function incrementAssetCount() public {
assetCount++; // Updates the state variable by incrementing the count
}
function incrementAssetCount() public {
assetCount++; // Updates the state variable by incrementing the count
}
function getAssetOwner(uint assetId) public view returns (address) {
return assetOwners[assetId]; // Returns the owner of the specified asset
}
This view function allows anyone to retrieve the owner of an asset using its ID without making any changes to the contract’s state, thus incurring no transaction costs.
Conclusion of Section 1.2:
The mechanics of state variables are fundamental to the operation and functionality of smart contracts in Solidity. Understanding how to declare, initialize, update, and read these variables is crucial for smart contract developers aiming to build efficient, secure, and functional decentralized applications.
Chapter 2: Writing to State Variables
Transactional Dynamics of Writing to State Variables
Understanding Transactions:
In the Ethereum blockchain, transactions play a pivotal role in modifying the state of a contract. When state variables within a Solidity contract are altered, these changes are made through transactions that are broadcasted to the network, mined, and subsequently included in a block. This process ensures the decentralized consensus, which is critical for the security and integrity of the blockchain.
Writing to State Variables:
Writing to a state variable requires a transaction. Each transaction involves a series of steps that ensure the change is valid and permanently recorded on the blockchain:
Transaction Initiation:
A user initiates a transaction by calling a function that modifies one or more state variables. This could be directly through a user interface connected to a wallet or programmatically via another contract.
Gas Estimation and Payment:
Every transaction requires gas, which is a fee that compensates for the computational energy required to process the transaction. Solidity developers need to be mindful of the gas cost when writing functions that update state variables, as inefficient code can lead to prohibitively high fees.
Mining and Consensus:
Once a transaction is submitted, it needs to be mined. Miners select transactions based on the gas price and other factors, execute the contract’s code, and validate the state changes. Successful mining includes the transaction in a new block, and the consensus mechanism of the blockchain ensures that all nodes update their ledgers to reflect this change.
Example of a State-Modifying Function:
function updateRecord(uint _recordId, string memory _newRecord) public {
records[_recordId] = _newRecord; // Modifies the state variable `records`
}
In this example, the function updateRecord modifies the records state variable. Each call to this function constitutes a transaction and changes the contract’s state on the blockchain. The transaction’s lifecycle—from initiation and gas payment to mining and ledger updating—ensures that the record update is securely and immutably stored.
Challenges and Considerations:
Scalability and Gas Costs: As the number of transactions increases, so does the demand for computational power, which can lead to higher gas costs. Developers must optimize contract interactions to minimize unnecessary state changes and manage transaction fees effectively.
Transaction Delays: Depending on network congestion and gas prices, transactions can experience delays, affecting the responsiveness of the contract. Developers might need to implement mechanisms to handle such delays gracefully in user interfaces.
Conclusion of Section:
The transactional dynamics associated with writing to state variables form the backbone of executing changes within Solidity smart contracts. Understanding these dynamics is essential for developers to create responsive, efficient, and secure applications on the Ethereum platform. By optimizing transaction interactions and managing gas costs, developers can enhance the performance and user experience of their decentralized applications.
Best Practices for Writing to State Variables in Solidity
Writing to state variables is a necessary but costly operation in terms of gas usage. Efficient management of these operations is crucial for optimizing performance and minimizing costs in smart contracts. Here are some best practices to consider when writing to state variables in Solidity:
1. Minimize State Changes:
Efficient Data Storage: Design your state variables to store data as efficiently as possible. Use appropriate data types that match the needs of your application. For example, using uint256 for storing large numbers or uint8 for smaller ranges can save gas by fitting the data storage to its necessary size.
Limit Write Operations: Wherever possible, reduce the frequency of write operations. Accumulate changes locally in memory or within a function and update the state variable in a single transaction.
2. Use Events for Non-Essential Data:
Logging Instead of Storing: For data that does not need to persist across function calls but should be traceable, consider using events. Events log data in transaction receipts rather than storing it on the blockchain, significantly reducing gas costs.
Example:
event RecordUpdated(uint indexed recordId, string oldData, string newData);
function updateRecord(uint recordId, string memory newData) public {
string memory oldData = records[recordId];
records[recordId] = newData;
emit RecordUpdated(recordId, oldData, newData);
}
3. Optimize Function Modifiers and Conditions:
Check Conditions Early: Implement checks early in your functions to fail fast and avoid unnecessary gas consumption for operations that won’t succeed. Use require or revert statements to validate conditions before making state changes.
Reuse Modifiers Sparingly: While function modifiers can help in reusing code for checks, they also add to the gas cost when used excessively. Only use modifiers for checks that are essential and common across multiple functions.
4. Batch Operations:
Group Similar Transactions: When multiple operations or transactions can be grouped, consider batching them to reduce the overhead of separate transaction costs. This is especially useful in functions that can naturally combine multiple similar tasks.
Example:
function updateMultipleRecords(uint[] memory recordIds, string[] memory newDatas) public {
for (uint i = 0; i < recordIds.length; i++) {
records[recordIds[i]] = newDatas[i];
}
}
5. Gas Optimization Techniques:
Solidity Compiler Settings: Utilize compiler optimizations to reduce the gas cost of deployed contracts. The Solidity compiler (solc) has settings that can optimize the contract bytecode to be more gas-efficient during execution.
Smart Contract Upgrades: Design your contracts with upgradeability in mind if they will require modifications in the future. This prevents the need for redeploying contracts which is often more costly.
Conclusion of Section 2.2:
Adhering to these best practices for writing to state variables can significantly enhance the efficiency and cost-effectiveness of your Solidity smart contracts. By carefully planning and implementing these strategies, developers can ensure that their contracts are not only robust and secure but also optimized for the unique economic environment of the Ethereum blockchain.
Chapter 3: Reading from State Variables
Cost Efficiency of Reading Operations:
Reading state variables in Solidity is a crucial operation, especially for dApp (decentralized application) interfaces that frequently need to display current contract states without modifying them. Understanding the cost-efficiency of these operations can significantly enhance the performance and user experience of blockchain applications.
Zero Transaction Costs for Reads:
Unlike writing operations that modify the blockchain state and incur gas fees, reading data from state variables does not require any gas if the read operation is external and does not alter the state. This is because read operations are executed locally on the node handling the request and do not require consensus from the network, thereby incurring no execution cost.
Using view and pure Functions:
Solidity provides two function types that are optimized for read operations:
view Functions: Functions that declare the view modifier promise not to modify the state. They are used to access state variables and return their values. These functions execute locally and do not change the contract’s data, thus do not require any gas when called externally.
pure Functions: Functions with the pure modifier are even more restrictive as they promise not to read from or modify the state. They typically perform calculations or return constants and are ideal for functions that need to compute data without any blockchain data interaction.
Example of Efficient Read Operations:
/// SPDX-License-Identifier: MIT
pragma solidity ^0.8.17;
contract DataReader {
uint public constant data = 42;
// A view function to read state
function readData() public view returns (uint) {
return data;
}
// A pure function to calculate values
function doubleData() public pure returns (uint) {
return 2 * 42; // Returns computed value without accessing state
}
}
In this example, readData is a view function that reads the value of a state variable without incurring any gas cost when called externally. doubleData is a pure function that computes a value, showing how computations can be performed off-chain to reduce costs and enhance efficiency.
Optimizing Client-Side Calls:
Developers can optimize dApp performance by handling read operations client-side wherever possible. This approach leverages the blockchain’s ability to serve reliable data without cost, enhancing the dApp’s responsiveness and scalability.
Strategic Use of Caching:
Caching frequently accessed data on the client side or within the dApp’s intermediate layers can significantly reduce the need for repeated read operations, thus improving efficiency and user experience. This strategy is particularly beneficial for data that changes infrequently but is read frequently.
Conclusion of Section 3.1:
The cost efficiency of reading operations in Solidity allows developers to design highly responsive and economical dApps. By understanding and utilizing view and pure functions appropriately, and by implementing client-side strategies such as caching, developers can ensure that their dApps are not only functional and secure but also optimally efficient in terms of both cost and resource usage.
Strategic Implementation of Read Functions:
Optimizing Data Retrieval:
In the development of smart contracts using Solidity, strategically implementing read functions is crucial for ensuring that data retrieval is efficient and effective. This optimization not only enhances the user experience by providing quick access to necessary data but also conserves resources by utilizing the blockchain’s capabilities judiciously.
1. Designing Read Functions for Maximum Efficiency:
Selective Data Exposure: When designing read functions, carefully consider which pieces of data need to be exposed externally. Use the public visibility modifier for state variables only when necessary, as Solidity automatically generates getter functions for these variables, which could lead to unnecessary data exposure and potential security risks.
Structured Data Returns: Structure the return values of your functions to provide exactly what is needed by the front end or other contracts, nothing more. This practice reduces the amount of data processed and transmitted, thereby improving performance and reducing latency.
Example of an Efficient Read Function:
contract OrderTracker {
struct Order {
uint id;
string details;
bool fulfilled;
}
mapping(uint => Order) private orders;
// Function to get order details if fulfilled
function getOrderDetails(uint orderId) public view returns (string memory) {
if (orders[orderId].fulfilled) {
return orders[orderId].details;
}
return “Order not fulfilled yet.”;
}
}
This example illustrates a view function that conditionally returns data based on the state of an order. By incorporating logical checks within the read function, it ensures that only relevant data is sent out, enhancing security and efficiency.
2. Leveraging view and pure Modifiers:
Correct Modifier Usage: Use the view modifier for functions that need to access the state but not modify it, and the pure modifier for functions that do not even read the state. This clarity in function design helps the Solidity compiler optimize the generated bytecode and reduces the cognitive load on developers and auditors by making function intentions explicit.
3. Caching Patterns for Frequent Reads:
Implement Caching: For data that is frequently read but rarely changes, consider implementing caching mechanisms at the application level. Caching can dramatically reduce the number of calls to the blockchain, thereby decreasing load and improving response times for users.
Smart Contract Events for Updates: Use events to notify front-end applications of state changes. Front-ends can listen to these events and update their local caches accordingly, which is particularly effective for dApps with high user interaction and data read requirements.
4. Error Handling and Data Validation:
Validate Inputs: Always validate inputs in your read functions to prevent errors and ensure that the returned data is accurate. Proper validation can prevent unnecessary calls and potential breakdowns in application logic.
Error Messaging: Provide clear error messages or default values in your read functions. This helps in debugging and ensures that the application handles unexpected or erroneous states gracefully.
Conclusion of Section 3.2:
The strategic implementation of read functions plays a pivotal role in the usability and efficiency of smart contracts. By carefully considering how data is accessed, optimized, and presented, developers can greatly enhance the functionality and responsiveness of their decentralized applications. This section has outlined key strategies and practices that can be adopted to refine data retrieval processes, ultimately leading to more sophisticated and user-friendly blockchain solutions.
Conclusion:
Understanding and implementing reading and writing operations to state variables are crucial for developing sophisticated and robust smart contracts on the Ethereum blockchain. This comprehensive analysis not only elevates developers’ proficiency in Solidity but also enhances the overall performance and security of decentralized applications. Through meticulous planning and strategic coding, developers can optimize resource utilization, making smart contracts both cost-effective and powerful.
Call to Action:
Leverage the insights from this study to refine your approach to smart contract development. Embrace the practices that promote efficiency and reliability to ensure your decentralized applications operate seamlessly and economically on the blockchain.
References
- Ethereum Foundation. (n.d.). Solidity Documentation. Retrieved from https://docs.soliditylang.org/en/v0.8.0/
- ConsenSys. (2021). Solidity Patterns. Retrieved from https://consensys.github.io/smart-contract-best-practices/solidity-patterns/
- OpenZeppelin. (n.d.). OpenZeppelin Contracts. Retrieved from https://docs.openzeppelin.com/contracts/4.x/
- Trail of Bits. (2019). Smart Contract Security Guidance. Retrieved from https://blog.trailofbits.com/2019/07/09/smart-contract-security-guidance/
- Nomic Foundation. (n.d.). Hardhat: Ethereum Development Environment. Retrieved from https://hardhat.org/
- DASP. (n.d.). Decentralized Application Security Project (DASP) Top 10. Retrieved from https://dasp.co/
- Chainlink. (n.d.). Chainlink Documentation. Retrieved from https://docs.chain.link/