Unveiling the Magic of Ether and Wei in Solidity Empowering Ethereum Development
Contents
- 1 Unveiling the Magic of Ether and Wei in Solidity Empowering Ethereum Development
- 1.1 Chapter 1: The Ethereal Essence of Ether and Wei
- 1.1.1 The Celestial Role of Ether
- 1.1.2 Ether as the Driving Force of the Ethereum Network:
- 1.1.3 Fuel for Transactions and Contract Interactions:
- 1.1.4 Incentive for Network Participants:
- 1.1.5 Governance and Network Upgrades:
- 1.1.6 Ether’s Economic Implications:
- 1.1.7 Capital Raising through Initial Coin Offerings (ICOs) and Token Sales:
- 1.1.8 Staking in Ethereum 2.0:
- 1.1.9 Medium of Exchange within Decentralized Finance (DeFi):
- 1.1.10 Cultural and Symbolic Significance:
- 1.1.11 Wei: The Subtle Dust of Ethereum
- 1.1.12 Fundamental Unit of Ether:
- 1.1.13 Technical and Operational Significance:
- 1.1.14 Granularity in Transactions:
- 1.1.15 Gas Calculations:
- 1.1.16 Programming with Wei in Solidity:
- 1.1.17 Cultural and Practical Implications:
- 1.1.18 Enabling Microtransactions:
- 1.1.19 Educational Tool:
- 1.2 Chapter 2: Solidity’s Conduits for Ether and Wei Manipulation
- 1.2.1 Interacting with Ether and Wei in Solidity:
- 1.2.2 Mastery of Microtransactions and Gas Calculations
- 1.2.3 Microtransactions Enabled by Wei:
- 1.2.4 Example of Microtransaction Implementation:
- 1.2.5 Gas Calculations and Optimization:
- 1.2.6 Gas Cost Calculation:
- 1.2.7 Conclusion of Section 2.2:
- 1.2.8 Chapter 3: Practical Applications and Implications
- 1.2.9 Enhancing dApp Functionality with Ether and Wei
- 1.2.10 Integrating Financial Operations:
- 1.2.11 Facilitating Varied Economic Models:
- 1.2.12 Implementing Micropayment Channels:
- 1.2.13 Example of a Micropayment Channel Using Wei:
- 1.2.14 Enhancing User Experience:
- 1.2.15 Conclusion of Section 3.1:
- 1.2.16 Educational Implications of Understanding Ether and Wei
- 1.2.17 Empowering Developers with Fundamental Knowledge:
- 1.2.18 Precision in Economic Transactions:
- 1.2.19 Example in Educational Content:
- 1.2.20 Enhancing Smart Contract Efficiency:
- 1.2.21 Fostering a Deeper Understanding of Blockchain Dynamics:
- 1.2.22 Preparing Developers for Advanced Blockchain Challenges:
- 1.2.23 Conclusion of Section 3.2:
- 1.2.24 Conclusion:
- 1.2.25 References
#EnterTheSmartContractSecuritySeries008
Unveiling the Magic of Ether and Wei in Solidity Empowering Ethereum Development
Abstract:
Venture into the mystical world of Ethereum and Solidity where Ether and Wei reign as fundamental units of value, fueling the Ethereum blockchain’s expansive ecosystem. This scholarly exposition delves deep into the intricacies of Ether and Wei, elucidating their pivotal role in crafting sophisticated smart contracts and enhancing the decentralized application landscape. By comprehending the nuanced relationship between these units, developers unlock new realms of innovation and efficiency in blockchain technology.
Introduction:
In the enchanting realm of Ethereum, the concepts of Ether and Wei represent more than mere currency—they embody the quintessential elements that power and facilitate the operational dynamics of smart contracts. As Ethereum continues to revolutionize decentralized applications (dApps), understanding these elemental units becomes indispensable for developers. This article embarks on a magical journey to explore the profound implications and functionalities of Ether and Wei within the Solidity programming environment.
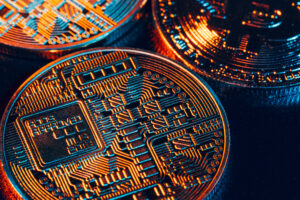
Unveiling the Magic of Ether and Wei in Solidity Empowering Ethereum Development
Chapter 1: The Ethereal Essence of Ether and Wei
The Celestial Role of Ether
Ether as the Driving Force of the Ethereum Network:
Ether is not just a unit of currency; it is the lifeblood that fuels the Ethereum ecosystem—a dynamic and expansive network supporting a myriad of decentralized applications (dApps). Its multifaceted roles include:
Fuel for Transactions and Contract Interactions:
Ether is used to pay for gas, the unit that measures the computational effort required to execute operations such as transactions, smart contract deployments, and contract interactions. This mechanism ensures that resources on the Ethereum network are used efficiently and prevents spamming of the network.
Incentive for Network Participants:
Ether serves as a powerful incentive for miners and validators who play a crucial role in maintaining the security and integrity of the Ethereum network. By rewarding these participants with Ether for validating transactions and blocks, the Ethereum blockchain ensures its operational continuity and security.
Governance and Network Upgrades:
Ether also plays a crucial role in the governance mechanisms of the Ethereum ecosystem. Holders of Ether can participate in decisions regarding network upgrades and changes, influencing the direction and functionality of the Ethereum platform through collective consensus.
Ether’s Economic Implications:
The economic role of Ether extends beyond simple transactional uses; it underpins a complex economy built around the Ethereum platform. Ether facilitates:
Capital Raising through Initial Coin Offerings (ICOs) and Token Sales:
As the primary currency on Ethereum, Ether is often used to raise capital for new projects and startups within the ecosystem. This is typically done through ICOs or token sales, where participants exchange Ether for new tokens built on Ethereum, driving forward innovation and development within the ecosystem.
Staking in Ethereum 2.0:
With the ongoing upgrade to Ethereum 2.0, Ether’s role is expanding to include staking. Users can lock up their Ether to participate in the network’s security as validators. This not only helps secure the network but also provides stakers with rewards in Ether, enhancing its function as an active investment vehicle.
Medium of Exchange within Decentralized Finance (DeFi):
Ether is widely used as a medium of exchange in DeFi applications, facilitating a wide range of financial services such as lending, borrowing, and yield farming. Its liquidity and interoperability make it a cornerstone asset in the DeFi space.
Cultural and Symbolic Significance:
Ether is often viewed as more than a cryptocurrency; it is a symbol of the transformative potential of blockchain technology. Its use in enabling decentralized and autonomous operations on a global scale highlights its symbolic value as a tool for financial and social innovation.
Wei: The Subtle Dust of Ethereum
Fundamental Unit of Ether:
Wei, the smallest denomination of Ether, serves as the fundamental unit within the Ethereum blockchain. Named after Wei Dai, a pioneer in the field of cryptocurrencies, this minuscule unit allows for precision and granularity in financial transactions on the Ethereum network. Understanding Wei is crucial for developers and users alike, as it enables the handling of microtransactions and precise gas calculations essential for optimizing smart contract operations.
Technical and Operational Significance:
Granularity in Transactions:
Wei offers the ability to conduct transactions involving very small amounts of Ether. This is particularly important in the context of decentralized applications (dApps) where transaction values can vary widely, and precise calculations are necessary to ensure fairness and accuracy in dealings.
Gas Calculations:
All transactions on Ethereum require gas, and gas costs are calculated in Wei. This ensures that even the smallest unit of work on Ethereum is quantifiable and payable, making Wei integral to the Ethereum’s operational framework. The granularity of Wei allows users to specify exactly how much they are willing to pay for a transaction, providing control and flexibility in resource allocation.
Programming with Wei in Solidity:
Solidity, Ethereum’s primary programming language, provides native support for expressing monetary values in Wei, ensuring that developers can easily integrate this unit into their code. Here’s an example illustrating how Wei is typically used in Solidity:
pragma solidity ^0.8.17;
contract MicroPayments {
// Function to deposit a small amount of Ether in Wei
function deposit() public payable {
require(msg.value == 10 wei, “Only exact 10 wei are accepted”);
}
// Function to convert Ether to Wei for precise operations
function convertToWei(uint etherAmount) public pure returns (uint) {
return etherAmount * 1 ether;
}
}
In this contract, the deposit function accepts a very small fixed amount of Ether, specifically 10 Wei, demonstrating the use of Wei for microtransactions. The convertToWei function provides a utility to convert Ether to Wei, facilitating precise financial operations.
Cultural and Practical Implications:
Enabling Microtransactions:
The use of Wei is particularly beneficial in applications that require microtransactions, such as pay-per-use services or minute financial exchanges in dApps. It allows the Ethereum network to accommodate a broader range of economic activities, expanding its utility beyond large-scale transactions.
Educational Tool:
For new developers and users entering the Ethereum ecosystem, understanding Wei is fundamental. It serves as an educational tool that aids in grasping the mechanics of Ethereum transactions and gas computations, fostering a deeper appreciation and mastery of the blockchain’s capabilities.
Chapter 2: Solidity’s Conduits for Ether and Wei Manipulation
Interacting with Ether and Wei in Solidity:
Solidity provides developers with the tools to manipulate these units effectively:
pragma solidity ^0.8.17;
contract EtherWeiHandling {
// Demonstrating Ether and Wei handling
uint public oneEtherInWei = 1 ether; // 1 Ether expressed in Wei
uint public oneWeiInEther = 1 wei; // The minimal unit of Ether
function convertEtherToWei(uint etherAmount) public pure returns (uint) {
return etherAmount * 1 ether; // Conversion from Ether to Wei
}
function convertWeiToEther(uint weiAmount) public pure returns (uint) {
return weiAmount / 1 ether; // Conversion from Wei to Ether
}
}
This contract exemplifies basic interactions with Ether and Wei, showcasing conversions that facilitate precise economic transactions within the blockchain.
Mastery of Microtransactions and Gas Calculations
Microtransactions Enabled by Wei:
In the intricate ecosystem of Ethereum, Wei enables microtransactions, which are essential for the expansive application of blockchain technology across various sectors. By allowing transactions that involve minuscule amounts of Ether, Wei supports economic models that are not feasible with larger currency units. This capability is particularly crucial in sectors like gaming, micro-insurance, and decentralized social media platforms, where small, frequent transactions form the core of user interactions.
Example of Microtransaction Implementation:
pragma solidity ^0.8.17;
contract TipJar {
// Accept tips in Wei
function tip() public payable {
require(msg.value > 0 && msg.value < 1 ether, “Only small tips accepted”);
}
// Withdraw tips in Ether
function withdrawTips() public {
payable(msg.sender).transfer(address(this).balance);
}
}
In this contract, TipJar allows users to give small tips, which are typically microtransactions. The function tip ensures that only amounts less than 1 Ether (expressed in Wei for precision) are accepted, promoting the use of microtransactions.
Gas Calculations and Optimization:
Understanding and optimizing for gas is paramount in developing efficient smart contracts. Each operation on the Ethereum network, from simple transactions to complex contract interactions, costs gas, and these costs are calculated in Wei.
Gas Cost Calculation:
Every transaction in Ethereum includes a gas limit and a gas price, both of which are denominated in Wei. The gas limit is the maximum amount of gas that the transaction can consume, and the gas price is the amount of Wei the sender is willing to pay per unit of gas. The total transaction fee is therefore the product of the gas used by the transaction and the gas price.
Optimizing Gas Usage:
Developers must write efficient Solidity code to minimize gas consumption. This includes optimizing loops, minimizing state changes, and using cheaper data storage options like events when appropriate. Additionally, the use of pure and view functions when querying blockchain state can significantly reduce gas costs since they don’t alter the blockchain state.
Example of Gas Optimization:
pragma solidity ^0.8.17;
contract GasSaver {
uint public result;
// Using pure function to perform calculations without costing gas
function multiply(uint x, uint y) public pure returns (uint) {
return x * y;
}
// Updating state variable minimally
function updateResult(uint _result) public {
if(_result != result) {
result = _result;
}
}
}
In this example, multiply is a pure function that performs a calculation without costing any gas for the computation per se when called externally. The updateResult function is designed to update the state variable result only if the new value differs from the current value, minimizing unnecessary gas consumption for state changes.
Conclusion of Section 2.2:
Mastering the nuances of Wei and gas calculations is essential for developing scalable and economically viable applications on Ethereum. By enabling microtransactions and optimizing for minimal gas usage, developers unlock the potential of Ethereum to support a vast array of business models and application scenarios, thereby driving the wider adoption and functionality of blockchain technology.
Chapter 3: Practical Applications and Implications
Enhancing dApp Functionality with Ether and Wei
Integrating Financial Operations:
The flexibility and precision provided by Ether and Wei are instrumental in enhancing the functionality of decentralized applications (dApps). These units allow developers to integrate complex financial operations directly into their applications, enabling a wide range of economic activities.
Facilitating Varied Economic Models:
Subscription Services: Ether and Wei can be used to handle subscription fees for services provided by dApps, allowing users to pay in small, regular amounts. This can be especially useful for content platforms, software as a service (SaaS) models, or any application requiring recurring payments.
In-App Purchases: For applications that offer digital goods or services, such as games or online marketplaces, the ability to conduct transactions in Wei enables users to make small, precise payments for specific purchases.
Implementing Micropayment Channels:
Wei’s granularity facilitates the creation of micropayment channels, which allow users to make numerous small transactions off-chain before settling the total amount on-chain. This significantly reduces transaction costs and network congestion, while still ensuring security through the Ethereum blockchain.
Example of a Micropayment Channel Using Wei:
pragma solidity ^0.8.17;
contract MicropaymentChannel {
address payable public receiver;
address public sender;
uint public expiration;
uint public amountDeposited;
constructor(address payable _receiver, uint duration) payable public {
sender = msg.sender;
receiver = _receiver;
expiration = block.timestamp + duration;
amountDeposited = msg.value; // Amount in Wei
}
function close(uint amount, bytes memory signature) public {
require(msg.sender == receiver, “Only receiver can close the channel.”);
require(isValidSignature(amount, signature), “Invalid signature.”);
require(amount <= amountDeposited, “Amount exceeds deposit.”);
receiver.transfer(amount);
selfdestruct(sender); // Sender gets back the remaining funds.
}
function extend(uint newExpiration) public {
require(msg.sender == sender, “Only sender can extend.”);
require(newExpiration > expiration, “New expiration must be later.”);
expiration = newExpiration;
}
function isValidSignature(uint amount, bytes memory signature) private view returns (bool) {
bytes32 message = prefixed(keccak256(abi.encodePacked(this, amount)));
return recoverSigner(message, signature) == sender;
}
// Add the prefixed and recoverSigner functions for signature verification
}
This contract example demonstrates how a micropayment channel can be implemented using Wei, enabling transactions that are cost-effective and efficient for both parties involved. The contract facilitates numerous small transactions off-chain with a final settlement on-chain, showcasing the flexibility and practicality of using Wei in real-world applications.
Enhancing User Experience:
Immediate Feedback and Interaction: By leveraging the fast and cost-effective characteristics of Wei for transactions, dApps can offer users immediate feedback and smoother interactions. This is crucial for user retention and satisfaction, as it enhances the overall responsiveness and usability of the application.
Conclusion of Section 3.1:
Ether and Wei dramatically enhance the functionality of dApps by enabling diverse economic transactions, micropayment channels, and improved user interactions. Their integration into dApps not only broadens the scope of what these applications can achieve but also deepens the engagement and utility for end-users. By understanding and utilizing these units effectively, developers can unlock the full potential of Ethereum to drive innovation and user adoption in the decentralized space.
Educational Implications of Understanding Ether and Wei
Empowering Developers with Fundamental Knowledge:
Understanding the distinctions and applications of Ether and Wei is crucial for any developer entering the Ethereum blockchain space. This foundational knowledge not only enhances technical proficiency but also equips developers with the necessary tools to design and implement sophisticated financial interactions within their decentralized applications (dApps).
Precision in Economic Transactions:
Accurate Calculations: Grasping the conversion between Ether and Wei allows developers to execute precise calculations necessary for handling transactions, setting gas prices, and managing smart contract functions that involve financial elements. This precision prevents common errors that could lead to significant financial discrepancies or vulnerabilities within the contract.
Example in Educational Content:
// Demonstrating the importance of precision in smart contract operations
contract FinancialCalculations {
uint public oneEtherInWei = 1 ether; // Shows conversion for educational purposes
// Function to calculate payment splits
function splitPayment(uint totalAmountInWei, uint numberOfRecipients) public pure returns (uint) {
return totalAmountInWei / numberOfRecipients;
}
}
This example can be used in educational settings to illustrate how understanding Wei can lead to more accurate financial programming in Solidity, emphasizing the importance of precise arithmetic operations.
Enhancing Smart Contract Efficiency:
Gas Optimization: Mastery of Ether and Wei directly contributes to optimizing gas costs. Developers who understand the fine-grained control over transactions provided by Wei can minimize expenses by optimizing the gas used in contract operations, a crucial skill in making dApps more accessible and sustainable.
Educational Workshops and Tutorials: Workshops focusing on gas optimization techniques provide practical scenarios where developers can apply their knowledge of Ether and Wei to reduce costs, thus making their applications more efficient and economical.
Fostering a Deeper Understanding of Blockchain Dynamics:
Comprehensive Blockchain Education: Knowledge of Ether and Wei extends beyond simple value transfer—it encompasses an understanding of Ethereum’s underlying mechanics, such as block validation, transaction fees, and the consensus process. Educational courses and resources that cover these topics help build a holistic view of blockchain technology.
Encouraging Innovation: With a solid grasp of how transactions and microtransactions are managed on the Ethereum network, developers can innovate more freely, experimenting with new types of financial interactions and incentive mechanisms within their projects.
Preparing Developers for Advanced Blockchain Challenges:
Advanced Courses and Certifications: As developers become proficient with the basic concepts of Ether and Wei, they can pursue advanced studies that delve into complex aspects of Ethereum development, including security practices, advanced economic models, and cross-chain integrations.
Community Engagement and Knowledge Sharing: Understanding the nuances of Ether and Wei prepares developers to contribute meaningfully to discussions and debates within the Ethereum community, enhancing collaborative projects and community-driven innovations.
Conclusion of Section 3.2:
The educational implications of understanding Ether and Wei are profound, affecting not only individual developer proficiency but also the broader Ethereum community’s capacity for innovation. By integrating this knowledge into educational programs, tutorials, and community discussions, the Ethereum ecosystem can continue to evolve and adapt to new challenges, paving the way for a future where decentralized applications flourish on a foundation of solid technical understanding and financial precision.
Conclusion:
The magical essence of Ether and Wei in the Ethereum ecosystem cannot be overstated. As fundamental units of value and operation, their effective management is crucial for any serious Ethereum developer. This exploration into their roles and functionalities aims to empower developers with the knowledge to harness these units creatively and efficiently, pushing the boundaries of what is possible in the world of decentralized applications.
References
- Ethereum Foundation. (n.d.). Solidity Documentation: Units and Globally Available Variables. Retrieved from https://docs.soliditylang.org/en/v0.8.0/units-and-global-variables.html
- ConsenSys. (2021). Ethereum Basics: Ether and Gas. Retrieved from https://consensys.net/knowledge-base/ethereum-basics/ether-gas/
- OpenZeppelin. (n.d.). OpenZeppelin Contracts. Retrieved from https://docs.openzeppelin.com/contracts/4.x/
- Trail of Bits. (2019). Smart Contract Security Guidance. Retrieved from https://blog.trailofbits.com/2019/07/09/smart-contract-security-guidance/
- Nomic Foundation. (n.d.). Hardhat: Ethereum Development Environment. Retrieved from https://hardhat.org/
- DASP. (n.d.). Decentralized Application Security Project (DASP) Top 10. Retrieved from https://dasp.co/
- Chainlink. (n.d.). Chainlink Documentation. Retrieved from https://docs.chain.link/
- Medium. (2020). Understanding Ether and Wei. Retrieved from https://medium.com/coinmonks/understanding-ether-and-wei-671f12d1a6b5