Solidity If/Else Statements: A Comprehensive Guide to Conditional Logic in Smart Contracts
Contents
- 1 Solidity If/Else Statements: A Comprehensive Guide to Conditional Logic in Smart Contracts
- 1.1 Chapter 1: Theoretical Framework of Conditional Logic in Solidity
- 1.1.1 1.1 Introduction to Conditional Logic:
- 1.1.2 1.2 The Role of If/Else Statements in Solidity:
- 1.1.3 1.3 Syntax and Structural Components:
- 1.1.4 If Statement: The simplest form of conditional, an if statement executes its code block if the condition evaluates to true.
- 1.1.5 1.4 Comparison Operators and Boolean Expressions:
- 1.1.6 1.5 Practical Considerations in Implementing Conditional Logic:
- 1.1.7 1.6 Advanced Conditional Constructs:
- 1.1.8 1.7 Theoretical Implications of Conditional Logic:
- 1.1.9 Conclusion of Chapter 1:
- 1.2 Chapter 2: Practical Application and Analysis
- 1.2.1 2.1 Applying If/Else Statements in Smart Contract Development:
- 1.2.2 2.2 Example Smart Contract with Detailed Analysis:
- 1.2.3 To demonstrate the practical usage of if/else statements, consider a smart contract designed to handle financial transactions with conditional validations:
- 1.2.4 Analysis of the Smart Contract:
- 1.2.5 2.3 Benefits of Using Conditional Logic in Smart Contracts:
- 1.2.6 2.4 Challenges and Considerations:
- 1.2.7 2.5 Case Study: Optimization of Conditional Statements:
- 1.2.8 Consider a scenario where conditional logic needs to be optimized for gas efficiency:
- 1.2.9 Conclusion of Chapter 2:
- 1.3 Chapter 3: Optimization and Best Practices
- 1.3.1 3.1 Optimization Techniques for Conditional Statements:
- 1.3.2 Example of Short-Circuit Optimization:
- 1.3.3 function isEligible(uint256 age, bool consent) public pure returns (bool) { return age >= 18 && consent; }
- 1.3.4 3.2 Best Practices for Implementing Conditional Logic:
- 1.3.5 Example of Clear Condition Usage:
- 1.3.6 if (isVerified == true) { // Code executes if isVerified explicitly true }
- 1.3.7 3.3 Advanced Conditional Patterns:
- 1.3.8 Example of Guard Clauses:
- 1.3.9 3.4 Testing and Validation:
- 1.3.10 Conclusion of Chapter 3:
- 1.3.11 Conclusion:
- 1.3.12 Sample Code Reference:
- 1.3.13 References
#EnterTheSmartContractSecuritySeries0011
Solidity If/Else Statements: A Comprehensive Guide to Conditional Logic in Smart Contracts
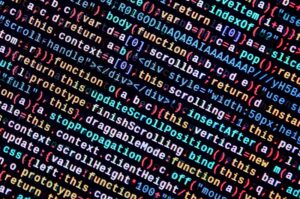
Solidity If/Else Statements: A Comprehensive Guide to Conditional Logic in Smart Contracts
Abstract:
This scholarly article delves into the utilization of if/else statements within Solidity, the primary programming language for Ethereum smart contracts. By exploring the syntax, operational logic, and practical implementations of conditional statements, this paper aims to elucidate their critical role in facilitating complex decision-making processes in blockchain-based applications.
Introduction:
Conditional statements form the backbone of logical structuring in computer programming, and Solidity is no exception. Within the Ethereum blockchain, these constructs enable developers to dictate the flow of execution based on dynamic conditions, thereby enhancing the functionality and reliability of decentralized applications (dApps).
Chapter 1: Theoretical Framework of Conditional Logic in Solidity
1.1 Introduction to Conditional Logic:
Conditional logic forms the core of decision-making in programming languages, and in Solidity, it plays a pivotal role in directing the execution flow of smart contracts based on dynamic conditions. This section introduces the fundamental concepts of conditional logic as applied within the Ethereum blockchain.
1.2 The Role of If/Else Statements in Solidity:
If/else statements in Solidity are crucial for controlling contract behaviors, enabling smart contracts to react differently under varying conditions. These statements assess boolean expressions — conditions that resolve either to true or false — and execute corresponding code blocks based on the evaluation.
1.3 Syntax and Structural Components:
If Statement: The simplest form of conditional, an if statement executes its code block if the condition evaluates to true.
if (condition) {
// Code executes if condition is true
}
Else If Statement: Used for multiple conditions that are mutually exclusive. Each condition is evaluated in order until one is true.
else if (anotherCondition) {
// Code executes if another condition is true
}
Else Statement: Captures all cases that do not meet any preceding conditions. It acts as a fallback within the conditional chain.
else {
// Code executes if no conditions above are met
}
1.4 Comparison Operators and Boolean Expressions:
Solidity supports standard comparison operators such as == (equality), != (inequality), < (less than), > (greater than), <= (less than or equal to), and >= (greater than or equal to). These operators are pivotal in formulating the conditions that drive if/else logic.
1.5 Practical Considerations in Implementing Conditional Logic:
Deterministic Outcomes: It is critical to ensure that conditions within smart contracts are deterministic, meaning they produce the same output for the same input at any given time, ensuring reliability and predictability in contract execution.
Gas Considerations: Since every operation in Ethereum costs gas, efficient use of conditional statements can help optimize the gas usage of smart contracts. Conditions should be structured to minimize computational overhead, especially in frequently executed functions.
1.6 Advanced Conditional Constructs:
Beyond simple if/else structures, Solidity allows for more complex conditional constructs, such as nested if statements and conditional loops, which can accommodate sophisticated decision-making processes within contracts. However, developers must handle these constructs carefully to maintain readability and avoid excessive gas costs.
1.7 Theoretical Implications of Conditional Logic:
The use of conditional statements in smart contracts introduces a layer of complexity in contract design but also significantly enhances the functionality and flexibility of blockchain applications. By enabling dynamic response mechanisms, if/else statements facilitate a broad range of financial, administrative, and logistical applications on the Ethereum network.
Conclusion of Chapter 1:
Understanding the theoretical underpinnings of conditional logic in Solidity is essential for any developer engaged in smart contract development. This chapter has laid the groundwork for appreciating how such logic not only dictates the operational flow of contracts but also impacts their efficiency and effectiveness in real-world applications.
Chapter 2: Practical Application and Analysis
2.1 Applying If/Else Statements in Smart Contract Development:
This section illustrates the practical application of if/else statements in Solidity through real-world scenarios, highlighting how these constructs govern the execution paths within smart contracts based on varying conditions.
2.2 Example Smart Contract with Detailed Analysis:
To demonstrate the practical usage of if/else statements, consider a smart contract designed to handle financial transactions with conditional validations:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.17;
contract PaymentProcessor {
address public owner;
uint256 public minimumDeposit = 1 ether;
constructor() {
owner = msg.sender;
}
function deposit() public payable {
if (msg.value < minimumDeposit) {
revert(“Deposit must be at least 1 ether”);
} else {
// Proceed with a higher-value deposit process
}
}
function updateMinimumDeposit(uint256 _newMinimum) public {
if (msg.sender != owner) {
revert(“Only the owner can update the minimum deposit”);
} else {
minimumDeposit = _newMinimum;
}
}
}
Analysis of the Smart Contract:
Function deposit: This function uses an if/else statement to check whether the deposit amount meets the minimum requirement. If the deposited amount is less than 1 ether, the transaction is reverted with an error message. This conditional check ensures that all deposits adhere to the contract’s financial rules.
Function updateMinimumDeposit: Here, the if/else statement controls access to a critical contract setting. Only the contract owner can change the minimum deposit amount, enhancing the security and integrity of the contract’s operational parameters.
2.3 Benefits of Using Conditional Logic in Smart Contracts:
Enhanced Control and Security: Conditional statements enable developers to enforce rules and constraints dynamically, significantly enhancing the contract’s security and operational reliability.
Flexibility in Execution: By using if/else statements, developers can create versatile smart contracts that adapt their behavior based on the context of interactions, user inputs, or external conditions.
2.4 Challenges and Considerations:
Complexity and Gas Costs: While if/else statements add functional richness to smart contracts, they can also introduce complexity and increase gas costs if not used judiciously. Optimizing the conditions and minimizing unnecessary checks is crucial for maintaining efficiency.
Testing and Debugging: Comprehensive testing is required to ensure that all conditional branches behave as expected under different scenarios. This includes unit testing and integration testing to cover all possible paths through the contract’s logic.
2.5 Case Study: Optimization of Conditional Statements:
Consider a scenario where conditional logic needs to be optimized for gas efficiency:
function checkEligibility(uint256 userAge) public pure returns (string memory) {
return userAge >= 18 ? “Eligible” : “Not Eligible”;
}
Ternary Operator: This example demonstrates the use of the ternary operator as a concise alternative to if/else for simple conditions, reducing the bytecode size and potentially lowering gas costs.
Conclusion of Chapter 2:
The practical application of if/else statements in Solidity provides developers with powerful tools for directing contract execution and enforcing business logic. This chapter has explored the nuanced application of these constructs, offering developers guidance on leveraging conditional logic to build more dynamic, secure, and efficient smart contracts.
Chapter 3: Optimization and Best Practices
3.1 Optimization Techniques for Conditional Statements:
This section delves into specific strategies that can be employed to optimize if/else statements in Solidity, aiming to improve both the gas efficiency and execution speed of smart contracts.
Minimize Condition Complexity: Simplify the conditions in if/else statements as much as possible to reduce the computational load. For instance, evaluate the most likely conditions first or consolidate multiple conditions when feasible.
Short-Circuit Evaluation: Leverage the properties of logical operators (&&, ||) that stop evaluating as soon as the outcome is determined. This can prevent unnecessary computation by arranging simpler or more likely to succeed conditions before more complex ones.
Example of Short-Circuit Optimization:
function isEligible(uint256 age, bool consent) public pure returns (bool) {
return age >= 18 && consent;
}
In this function, if age is less than 18, Solidity doesn’t evaluate consent, saving gas if the age condition fails.
3.2 Best Practices for Implementing Conditional Logic:
Adhering to best practices in the use of if/else statements ensures that the smart contract code is not only efficient but also maintainable and secure.
Avoid Deep Nesting: Deeply nested if/else statements can make the code hard to read and maintain. Refactor complex conditional logic into separate functions or consider using other control structures like switch or lookup tables if applicable.
Use Explicit Conditions: Be explicit in conditions to enhance readability and prevent bugs. For instance, use == true or == false for boolean comparisons rather than relying on implicit type conversion.
Example of Clear Condition Usage:
if (isVerified == true) {
// Code executes if isVerified explicitly true
}
3.3 Advanced Conditional Patterns:
Explore advanced patterns and idioms that can lead to more efficient and clearer conditional logic in Solidity.
Guard Clauses: Use early returns (guard clauses) to handle edge cases or invalid conditions upfront. This reduces nesting and makes the main logic of the function clearer and more linear.
State Machine Implementation: In contracts with complex state-dependent behavior, model the contract’s states explicitly using enums and switch-like patterns for clarity and to ensure conditions and transitions are well-managed.
Example of Guard Clauses:
function withdraw(uint256 amount) public {
require(amount > 0, “Amount must be positive”);
if (balances[msg.sender] < amount) {
revert(“Insufficient balance”);
}
balances[msg.sender] -= amount;
emit Withdrawal(msg.sender, amount);
}
In this withdrawal function, guard clauses are used for validation before proceeding with the balance deduction.
3.4 Testing and Validation:
Comprehensive testing is essential to ensure that conditional logic behaves as expected under all circumstances.
Unit Tests: Write extensive unit tests for each logical branch in your conditional statements to verify behavior under various conditions.
Integration Tests: Conduct integration tests to see how your contract’s conditional logic interacts with other contracts and on-chain services.
Conclusion of Chapter 3:
Effective management and optimization of conditional statements are crucial for developing high-quality smart contracts in Solidity. This chapter has provided a detailed exploration of optimization strategies, best practices, and advanced patterns that developers can utilize to ensure their contracts are efficient, secure, and maintainable.
Conclusion:
The adept use of if/else statements in Solidity is essential for developing sophisticated and secure smart contracts on the Ethereum blockchain. This guide has provided a comprehensive understanding of conditional logic’s theoretical basis, practical implementation, and best practices for optimization.
Sample Code Reference:
The code snippets provided throughout the article serve as practical examples to help developers implement effective conditional logic in their smart contracts.