Unlocking Ethereum ABI Harnessing the Power of abi.decode in Solidity
Contents
- 1 Unlocking Ethereum ABI Harnessing the Power of abi.decode in Solidity
- 1.1 Introduction to ABI Decoding in Solidity
- 1.2 Understanding ABI Decoding
- 1.3 Practical Applications of abi.decode
- 1.4 Best Practices for Using abi.decode
- 1.5 Security Considerations
- 1.6 Conclusion
- 1.7 Resources on Ethereum ABI and abi.decode
#EnterTheSmartContractSecuritySeries0039
Unlocking Ethereum ABI Harnessing the Power of abi.decode in Solidity
Introduction to ABI Decoding in Solidity
Solidity, the programming language for developing smart contracts on the Ethereum blockchain, offers a rich set of tools for data encoding and decoding. One of the most essential functions for interacting with encoded data is abi.decode
. Understanding how to effectively use abi.decode
is crucial for developers looking to build sophisticated and interoperable decentralized applications (dApps).
In this article, we will explore the intricacies of ABI decoding in Solidity, delve into its practical applications, and discuss best practices for leveraging this powerful function.
Understanding ABI Decoding
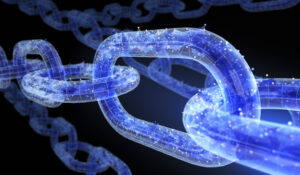
Unlocking Ethereum ABI Harnessing the Power of abi.decode in Solidit
What is ABI Decoding?
ABI (Application Binary Interface) decoding is the process of interpreting encoded data back into its original data types. This is essential for extracting meaningful information from the byte data returned by smart contract functions or passed through external calls.
The abi.decode Function
The abi.decode
function in Solidity is used to decode bytes data into specified data types. It is often used to handle the return data from function calls or to process incoming data in a contract.
Basic Syntax of abi.decode
The basic syntax for using abi.decode
is as follows:
(bytes memory encodedData) = /* some encoded data */;
(ReturnType1, ReturnType2, …) = abi.decode(encodedData, (ReturnType1, ReturnType2, …));
Practical Applications of abi.decode
Decoding Function Return Values
One of the primary uses of abi.decode
is to decode the return values of function calls, especially when dealing with low-level calls or interactions with external contracts.
Example: Decoding a Function’s Return Value
contract ExternalContract {
function getData() public pure returns (uint256, string memory) {
return (42, “Hello, World!”);
}
}
contract MyContract {
function callExternalFunction(address externalAddress) public returns (uint256, string memory) {
(bool success, bytes memory data) = externalAddress.call(
abi.encodeWithSignature(“getData()”)
);
require(success, “Call failed”);
(uint256 number, string memory text) = abi.decode(data, (uint256, string));
return (number, text);
}
}
In this example, MyContract
calls getData
on ExternalContract
, and uses abi.decode
to extract the uint256
and string
return values from the encoded data.
Processing Incoming Data
Contracts can receive encoded data that needs to be decoded for further processing. This is common in complex dApps where contracts communicate using encoded messages.
Example: Decoding Incoming Data
contract DataProcessor {
event DataDecoded(uint256 number, address addr);
function processData(bytes memory data) public {
(uint256 number, address addr) = abi.decode(data, (uint256, address));
emit DataDecoded(number, addr);
}
}
In this example, DataProcessor
decodes incoming data to extract a uint256
and an address
, then emits an event with the decoded values.
Handling Complex Data Structures
Solidity supports decoding complex data structures like arrays and structs, allowing for sophisticated data handling within smart contracts.
Example: Decoding an Array
contract ArrayDecoder {
event ArrayDecoded(uint256[] numbers);
function decodeArray(bytes memory data) public {
uint256[] memory numbers = abi.decode(data, (uint256[]));
emit ArrayDecoded(numbers);
}
}
This example demonstrates how to decode an encoded array of uint256
values and emit an event with the decoded array.
Working with Dynamic Types
Decoding dynamic types like strings and bytes requires special attention, as their lengths are not fixed and must be determined from the encoded data.
Example: Decoding Dynamic Types
contract DynamicTypeDecoder {
event StringDecoded(string decodedString);
function decodeString(bytes memory data) public {
string memory decodedString = abi.decode(data, (string));
emit StringDecoded(decodedString);
}
}
In this example, DynamicTypeDecoder
decodes an encoded string and emits an event with the decoded value.
Best Practices for Using abi.decode
Ensure Data Compatibility
Ensure that the data being decoded is compatible with the expected data types. Misalignment in types or data structure can lead to decoding errors and unexpected behavior.
Validate Decoded Data
Always validate the decoded data to ensure it meets the expected format and constraints. This helps maintain the integrity and security of your smart contract.
Minimize External Dependencies
Minimize dependencies on external data sources when possible. If external data must be used, ensure it is validated and sanitized before decoding.
Optimize Gas Usage
Decoding complex data structures can be gas-intensive. Optimize the encoding and decoding processes to minimize gas costs, especially for frequently called functions.
Security Considerations
Handle Malicious Data
Be cautious of maliciously crafted data that can exploit vulnerabilities in your decoding logic. Implement thorough input validation and error handling to mitigate such risks.
Prevent Reentrancy Attacks
When decoding data that involves external calls, be aware of reentrancy risks. Use the Checks-Effects-Interactions pattern to prevent reentrancy attacks.
Secure Data Storage
Ensure that decoded data is securely stored and handled within your contract. Avoid exposing sensitive information through poorly managed storage mechanisms.
Conclusion
The abi.decode
function in Solidity is a powerful tool for interpreting encoded data, enabling sophisticated data handling and interaction between smart contracts. By mastering ABI decoding and adhering to best practices, developers can build more robust, efficient, and secure dApps on the Ethereum blockchain.
Understanding the various applications of abi.decode
, from decoding function return values to processing complex data structures, will enhance your Solidity development skills. Whether you are interacting with external contracts, handling incoming data, or managing dynamic types, leveraging the power of ABI decoding is essential for advanced Solidity development.
By following these guidelines and being mindful of security considerations, you can harness the full potential of abi.decode
, creating sophisticated and interoperable decentralized applications.
Resources on Ethereum ABI and abi.decode
Official Documentation and Technical Guides
- Solidity Documentation: Offers detailed information about the Solidity language and ABI handling, including the use of
abi.decode
.
Educational Platforms and Courses
- CryptoZombies: An interactive coding platform that teaches Ethereum and Solidity development through building a game, including lessons on interacting with the Ethereum ABI.
- Ethereum and Solidity: The Complete Developer’s Guide (Udemy): A course that covers all aspects of Ethereum development, including smart contracts and ABI interactions.
Blogs and Technical Articles
- Ethereum Foundation Blog: Provides updates and tutorials directly from the Ethereum Foundation, which sometimes include detailed explanations of ABI specifics.
- Medium Articles on Ethereum Development: Various articles that discuss the nuances of Ethereum ABI and how to use
abi.decode
effectively in Solidity.
Forums and Community Discussions
- Ethereum Stack Exchange: A robust platform for asking questions and sharing insights about Ethereum, including detailed discussions on ABI and its functions in Solidity.
Tools for Development and Testing
- Remix IDE: An open-source tool that allows you to write, deploy, and test Solidity contracts, which is useful for experimenting with
abi.decode
and understanding its impact. - Ethers.js and Web3.js: Libraries that facilitate interaction with the Ethereum Blockchain, including ABI encoding and decoding, which can provide practical context to
abi.decode
usage in Solidity.