Reentrancy Vulnerability
Reentrancy is a concept that has garnered significant attention in the field of computer science, particularly within the realms of concurrent programming and blockchain development. This blog post delves into the intricacies of reentrancy, its implications, and its relevance in modern computing environments.
Introduction to Reentrancy
Reentrancy refers to the ability of a function to be interrupted in the middle of its execution and safely called again (“re-entered”) before the previous executions are complete. This capability is crucial in environments where functions need to be executed concurrently, ensuring that each invocation can proceed without interfering with others.
Historical Context
The concept of reentrancy emerged from the need to handle concurrency in early computing systems. As computers evolved from single-threaded to multi-threaded architectures, the necessity for functions to be safely reentrant became apparent. This was particularly vital in operating systems and real-time applications where interrupt handling and multitasking were common.
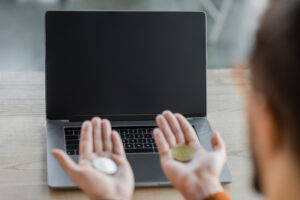
Reentrancy Vulnerability
Reentrancy in Concurrent Programming
In concurrent programming, reentrancy plays a pivotal role. Functions in a concurrent system may be invoked simultaneously by multiple threads. A non-reentrant function could lead to race conditions, data corruption, and unpredictable behavior.
Characteristics of Reentrant Functions
A function is considered reentrant if:
- It does not hold static or global data between invocations.
- It does not modify itself or its own code.
- It uses local variables instead of shared variables.
- It does not rely on non-reentrant functions.
Example of a Reentrant Function
int factorial(int n) {
if (n == 0) return 1;
else return n * factorial(n – 1);
}
In this example, factorial
is reentrant because it only uses local variables and does not depend on any external state.
Example of a Non-Reentrant Function
int counter = 0;
void increment() {
counter++;
}
Here, increment
is not reentrant because it modifies the global variable counter
.
Reentrancy in Blockchain Development
Reentrancy has become a critical concern in blockchain development, particularly with the rise of smart contracts. A famous example highlighting the risks associated with non-reentrant functions is the DAO attack on Ethereum.
The DAO Attack
The Decentralized Autonomous Organization (DAO) attack in 2016 exploited a reentrancy vulnerability in a smart contract. The attacker repeatedly called a function that transferred Ether before the contract could update its balance, allowing them to drain funds continuously.
Preventing Reentrancy Attacks
To prevent such vulnerabilities, developers can:
- Use the “Checks-Effects-Interactions” pattern.
- Implement reentrancy guards using mutexes or the
nonReentrant
modifier in Solidity.
Checks-Effects-Interactions Pattern
This pattern involves:
- Checking if the function can proceed (e.g., verifying sufficient balance).
- Updating the state to reflect the changes.
- Interacting with external contracts or transferring funds.
function withdraw(uint _amount) public nonReentrant {
require(balances[msg.sender] >= _amount, “Insufficient balance”);
balances[msg.sender] -= _amount;
msg.sender.transfer(_amount);
}
In this example, state changes are made before any external calls, preventing reentrancy.
Practical Applications and Implications
Real-Time Systems
In real-time systems, reentrancy is crucial for handling interrupts. Interrupt service routines (ISRs) must be reentrant to ensure that they can be executed at any time, even if the system is already handling an interrupt.
Embedded Systems
Embedded systems, often operating under stringent timing constraints, benefit from reentrant functions to maintain reliability and predictability.
Multi-Threaded Applications
For multi-threaded applications, reentrancy ensures that functions can be safely called by multiple threads simultaneously, enhancing performance and avoiding data corruption.
Conclusion
Reentrancy is a fundamental concept in both concurrent programming and blockchain development, essential for ensuring safe and predictable function execution in multi-threaded environments and preventing security vulnerabilities in smart contracts. Understanding and implementing reentrant functions is crucial for developers aiming to create robust and secure software systems.
Source
- DApp World – Understanding Reentrancy Attack This guide provides an overview of reentrancy attacks, a real-world example with the DAO hack, and preventive measures. It includes a sample vulnerable contract and attack contract. Read more here.
- Blockchain Magazine – Understanding Reentrancy Attacks and Top 10 Methods of Preventing Them This article outlines the best practices for preventing reentrancy attacks, including rigorous code audits, continuous testing, and the use of reentrancy guards and secure coding patterns. Read more here.
- Chainlink Blog – Reentrancy Attacks and The DAO Hack Explained This resource explains the reentrancy attack in detail, using the DAO hack as a case study. It includes a code walkthrough of the vulnerability and the attack. Read more here.