Ethereum Arithmetic Issues Vulnerability
Arithmetic issues in Ethereum smart contracts can lead to significant vulnerabilities and financial losses. This blog post provides an in-depth analysis of arithmetic problems in Ethereum, exploring their causes, implications, and strategies for prevention. The goal is to offer a comprehensive understanding suitable for a doctoral thesis level of detail.
Introduction to Arithmetic Issues in Ethereum
Ethereum, a decentralized platform that enables the execution of smart contracts, operates using the Solidity programming language. Arithmetic operations in Solidity, like addition, subtraction, multiplication, and division, are fundamental to many smart contracts. However, these operations can introduce critical vulnerabilities if not handled correctly.
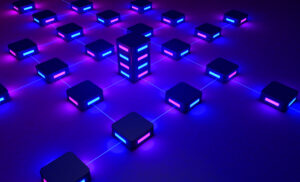
Arithmetic Issues in Ethereum
Importance of Arithmetic Operations
Arithmetic operations are central to many aspects of smart contracts, including:
- Managing balances and transactions
- Calculating fees and rewards
- Implementing token economics
Common Arithmetic Issues
The primary arithmetic issues in Ethereum include:
- Overflow and underflow
- Division by zero
- Precision loss
Overflow and Underflow
Overflow and underflow occur when an arithmetic operation exceeds the storage capacity of a data type.
Understanding Overflow and Underflow
- Overflow: Occurs when a value exceeds the maximum limit of its data type.
- Underflow: Occurs when a value goes below the minimum limit of its data type.
Example of Overflow
uint8 max = 255;
uint8 overflow = max + 1; // Results in 0 due to overflow
Example of Underflow
uint8 underflow = min – 1; // Results in 255 due to underflow
Real-World Example: The BatchOverflow Vulnerability
In April 2018, the BatchOverflow vulnerability affected several ERC-20 tokens. It allowed attackers to create an astronomical amount of tokens due to an unchecked overflow in the batchTransfer
function.
Code Example of Vulnerable Function
function batchTransfer(address[] _receivers, uint256 _value) public {
uint count = _receivers.length;
uint256 amount = uint256(count) * _value;
require(balances[msg.sender] >= amount);
balances[msg.sender] -= amount;
for (uint i = 0; i < count; i++) {
balances[_receivers[i]] += _value;
}
}
Here, uint256 amount = uint256(count) * _value
could overflow, allowing the transfer of an unintended number of tokens.
Preventing Overflow and Underflow
To prevent these issues, developers can:
- Use SafeMath Library: OpenZeppelin’s SafeMath library provides safe arithmetic operations that revert on overflow and underflow.
using SafeMath for uint256;
uint256 safeAmount = count.mul(_value);
Check Inputs: Validate inputs before performing arithmetic operations.
require(_value <= MAX_VALUE);
Division by Zero
Division by zero is another critical issue that can cause a smart contract to revert or exhibit undefined behavior.
Understanding Division by Zero
Division by zero occurs when a contract attempts to divide a number by zero, which is mathematically undefined and causes the EVM (Ethereum Virtual Machine) to throw an error.
Example of Division by Zero
uint256 result = 10 / denominator; // Causes a revert
Preventing Division by Zero
To prevent division by zero:
- Check Denominators: Ensure that the denominator is not zero before performing division.
require(denominator != 0, “Denominator cannot be zero”);
Use SafeMath: The SafeMath library provides a div
function that includes a zero check.
Precision Loss
Precision loss occurs in arithmetic operations involving fixed-point numbers, which are common in financial calculations.
Understanding Precision Loss
Precision loss happens when the result of an arithmetic operation is rounded, truncated, or otherwise altered due to the limitations of fixed-point arithmetic.
Example of Precision Loss
uint256 numerator = 10;
uint256 denominator = 3;
uint256 result = numerator / denominator; // Results in 3, losing the fractional part
Preventing Precision Loss
To mitigate precision loss:
- Use Higher Precision: Perform calculations using higher precision and adjust the result as necessary.
uint256 numerator = 10 * 10**18;
uint256 denominator = 3;
uint256 result = numerator / denominator; // Results in 3.3333… with higher precision
Fixed-Point Libraries: Utilize libraries designed for fixed-point arithmetic.
uint256 fixedResult = FixedPoint.div(numerator, denominator);
Advanced Strategies for Preventing Arithmetic Issues
Formal Verification
Formal verification involves mathematically proving the correctness of smart contracts. This technique can be used to ensure that arithmetic operations behave as expected under all possible conditions.
Example of Formal Verification Tool
- Solidity SMTChecker: A tool integrated into the Solidity compiler that uses formal methods to detect arithmetic issues.
pragma experimental SMTChecker;
contract SafeArithmetic {
function add(uint256 a, uint256 b) public pure returns (uint256) {
return a + b;
}
}
Static Analysis Tools
Static analysis tools analyze the code without executing it to detect potential vulnerabilities, including arithmetic issues.
Example of Static Analysis Tool
- MythX: A popular security analysis tool for Ethereum smart contracts.
pragma solidity ^0.8.0;
import “@openzeppelin/contracts/utils/math/SafeMath.sol”;
contract SecureContract {
using SafeMath for uint256;
function safeAdd(uint256 a, uint256 b) public pure returns (uint256) {
return a.add(b);
}
}
Audits and Code Reviews
Regular audits and peer code reviews can help identify and mitigate arithmetic issues before deploying smart contracts.
Conclusion
Arithmetic issues in Ethereum smart contracts pose significant risks, including overflow, underflow, division by zero, and precision loss. These vulnerabilities can lead to financial losses and undermine the integrity of decentralized applications. By understanding these issues and implementing preventive measures such as using the SafeMath library, validating inputs, leveraging formal verification, and conducting thorough code reviews, developers can create more secure and reliable smart contracts.
Source
- Vulnerability Scanners for Ethereum Smart Contracts: This study evaluates the performance of various vulnerability scanners and highlights common issues such as integer overflows and underflows. The analysis helps in understanding how these vulnerabilities can be detected and mitigated. Read more here.
- QuickNode: This resource provides a detailed discussion on arithmetic issues, including examples of overflow and underflow vulnerabilities and best practices for preventing them. Read more here.
- ImmuneBytes: This article explains specific arithmetic vulnerabilities with code examples and discusses real-world impacts and mitigation strategies. Read more here.