Ethereum Front-Running Vulnerability
Front-running is a critical issue in Ethereum and other blockchain ecosystems, where malicious actors exploit the transparency and speed of transactions to gain unfair advantages. This comprehensive blog post examines the nature of front-running in Ethereum, its various forms, real-world examples, and best practices for prevention, providing a detailed analysis suitable for a doctoral thesis.
Introduction to Front-Running
Front-running occurs when an attacker intercepts and preemptively executes a transaction based on prior knowledge of a pending transaction. This practice leverages the public and transparent nature of blockchain transactions to manipulate outcomes for financial gain.
Importance of Understanding Front-Running
Front-running poses significant risks to the integrity and fairness of decentralized applications (DApps) and decentralized finance (DeFi) protocols, leading to:
- Financial losses for legitimate users.
- Market manipulation.
- Erosion of trust in blockchain systems.
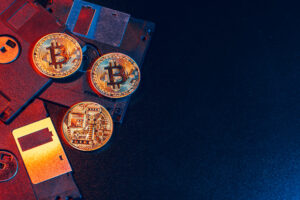
Front-Running in Ethereum: An In-Depth Analysis
Types of Front-Running Attacks
Front-running in Ethereum can be classified into various types, including:
- Transaction Ordering Dependence (TOD)
- Displacement
- Insertion
- Suppression
Transaction Ordering Dependence (TOD)
TOD, also known as miner extractable value (MEV), occurs when miners reorder transactions within a block to maximize their profit. By prioritizing transactions with higher fees or those that benefit the miner directly, miners can manipulate the outcome of transactions.
Example of TOD
A miner observes a high-value trade in a decentralized exchange (DEX) and inserts their own trade before it, causing the price to shift and profiting from the price difference.
Displacement
Displacement front-running involves submitting a transaction with a higher gas price to replace an existing pending transaction. The attacker displaces the original transaction to execute their own first.
Example of Displacement
A user submits a transaction to buy tokens at a low price. An attacker sees this and submits the same transaction with a higher gas fee, ensuring their transaction is mined first and the original transaction fails or executes at a worse price.
Insertion
Insertion front-running occurs when an attacker inserts their transaction between two dependent transactions. This can disrupt the sequence and lead to financial gain for the attacker.
Example of Insertion
A user submits a transaction to approve and transfer tokens. An attacker inserts a transaction to manipulate the token price between the approval and transfer, profiting from the price manipulation.
Suppression
Suppression involves submitting transactions to delay or prevent other transactions from being mined. This can be used to prevent market orders or liquidations from being executed.
Example of Suppression
An attacker submits multiple low-value transactions with high gas fees to fill the block, delaying the execution of a liquidation transaction in a lending protocol, allowing the attacker to profit from the delay.
Real-World Examples of Front-Running
Uniswap Front-Running
Uniswap, a popular decentralized exchange, has been a frequent target of front-running attacks. Attackers monitor the mempool (a pool of pending transactions) for large trades and submit their own transactions with higher gas fees to profit from price changes.
Bancor Network Front-Running
Bancor, another decentralized exchange, faced front-running issues where attackers exploited transaction ordering to manipulate token prices. This led to significant financial losses for legitimate users and highlighted the need for robust front-running mitigation strategies.
Preventing Front-Running Attacks
Increasing Transaction Privacy
One effective approach to prevent front-running is to increase the privacy of transactions. By making transaction details less visible, attackers cannot preemptively execute malicious transactions.
Zero-Knowledge Proofs
Zero-knowledge proofs (ZKPs) allow transactions to be verified without revealing their details. Integrating ZKPs in Ethereum can enhance privacy and reduce front-running risks.
Example of ZKP Integration
// Simplified example of a ZKP-based function
function verifyTransaction(bytes memory proof) public view returns (bool) {
// Verify the proof without revealing transaction details
return verifyProof(proof);
}
Commit-Reveal Schemes
Commit-reveal schemes involve a two-phase process where users first commit to a transaction without revealing its details and then reveal the transaction in a later phase. This method can mitigate front-running by hiding transaction details until the reveal phase.
Example of Commit-Reveal Scheme
contract CommitReveal {
struct Commit {
bytes32 commitment;
bool revealed;
}
mapping(address => Commit) public commits;
uint256 public revealPeriod;
constructor(uint256 _revealPeriod) {
revealPeriod = _revealPeriod;
}
function commit(bytes32 _commitment) public {
commits[msg.sender] = Commit(_commitment, false);
}
function reveal(uint256 _value, bytes32 _nonce) public {
require(keccak256(abi.encodePacked(_value, _nonce)) == commits[msg.sender].commitment, “Invalid commitment”);
commits[msg.sender].revealed = true;
// Handle the revealed value
}
}
Decentralized Batch Auctions
Decentralized batch auctions aggregate multiple orders and execute them in batches, reducing the ability of attackers to front-run individual transactions. This method can help level the playing field and ensure fair execution.
Example of Batch Auction
contract BatchAuction {
struct Order {
address user;
uint256 amount;
}
Order[] public orders;
function placeOrder(uint256 amount) public {
orders.push(Order(msg.sender, amount));
}
function executeBatch() public {
// Execute all orders in the batch
for (uint256 i = 0; i < orders.length; i++) {
// Process order
}
// Clear orders array
delete orders;
}
}
Time-Locked Transactions
Time-locked transactions introduce a delay between transaction submission and execution, giving users time to verify and cancel transactions if suspicious activity is detected.
Example of Time-Locked Transaction
contract TimeLock {
struct Transaction {
address user;
uint256 amount;
uint256 timestamp;
}
mapping(address => Transaction) public transactions;
uint256 public timeLockPeriod;
constructor(uint256 _timeLockPeriod) {
timeLockPeriod = _timeLockPeriod;
}
function submitTransaction(uint256 amount) public {
transactions[msg.sender] = Transaction(msg.sender, amount, block.timestamp);
}
function executeTransaction() public {
require(block.timestamp >= transactions[msg.sender].timestamp + timeLockPeriod, “Transaction is time-locked”);
// Execute transaction logic
}
}
Layer 2 Solutions
Layer 2 solutions, such as rollups and state channels, can reduce the impact of front-running by processing transactions off-chain and submitting aggregated results to the Ethereum mainnet.
Example of Layer 2 Solution
contract Layer2 {
// Simplified example of a Layer 2 contract
function processOffChainTransactions(bytes[] memory transactions) public {
// Process transactions off-chain and submit results
}
}
Conclusion
Front-running in Ethereum presents significant challenges to the security and fairness of decentralized applications. By understanding the various forms of front-running attacks and implementing best practices such as increasing transaction privacy, using commit-reveal schemes, decentralized batch auctions, time-locked transactions, and Layer 2 solutions, developers can mitigate these risks and enhance the integrity of the Ethereum ecosystem.
Source
- Flashbots—Frontrunning the MEV Crisis: This resource from Flashbots discusses the existential risks and negative externalities posed by Maximal Extractable Value (MEV) on Ethereum. It provides insights into how Flashbots aims to mitigate these risks through transparency, democratizing MEV extraction, and distributing benefits across the ecosystem. The resource emphasizes the need for a fair and open ecosystem for MEV extraction to preserve Ethereum’s core values. Read more here.
- MEV Explore by Flashbots: This site provides a comprehensive FAQ and explores the mechanisms of MEV, including front-running. It details how miners and validators utilize MEV strategies such as front-running, liquidations, and sandwich attacks to capture additional value. The resource also covers the implications of Ethereum’s shift to Proof-of-Stake and the introduction of Protocol/Builder Separation (PBS). Read more here.
- Ethereum Smart Contract Best Practices – ConsenSys: This guide from ConsenSys covers best practices for mitigating front-running attacks in Ethereum smart contracts. It includes specific recommendations for token contracts, highlighting how certain functions like
approve()
can be exploited through front-running. The resource provides practical advice on securing smart contracts against these vulnerabilities. Read more here. - Arbitrage and Frontrunning in DeFi by Bitcoin Suisse: This article explains how front-running and arbitrage work in decentralized finance (DeFi). It provides examples of front-running in action, detailing how bots scan the mempool for large orders and submit competing transactions with higher gas fees to get mined first. The resource offers a clear understanding of the dynamics and challenges associated with front-running in DeFi. Read more here.
- What are MEV and MEV Bots? by OpenPool: This blog post discusses MEV and the role of bots in front-running transactions on Ethereum. It covers how MEV strategies have evolved with Ethereum’s transition to Proof-of-Stake and the impact on transaction fees and network congestion. The article provides a historical perspective on MEV and its future implications. Read more here.