Ethereum Short Address Attack Vulnerability
The Short Address Attack is a critical vulnerability in Ethereum smart contracts, where malicious actors exploit discrepancies in how Ethereum handles addresses of varying lengths. This comprehensive blog post explores the nature of short address attacks, their implications, real-world examples, and best practices for prevention, providing a detailed analysis suitable for a doctoral thesis.
Introduction to Short Address Attack
Ethereum, as a decentralized platform, facilitates the execution of smart contracts using the Solidity programming language. Solidity enforces strict address lengths, typically 20 bytes (40 hexadecimal characters). A Short Address Attack takes advantage of how certain Ethereum clients handle address lengths that are shorter than expected, leading to erroneous execution of smart contracts.
Importance of Understanding Short Address Attacks
Solidity Short address attacks pose significant risks, including:
- Financial losses due to misdirected funds.
- Compromised integrity of decentralized applications (DApps).
- Erosion of trust in smart contract security.
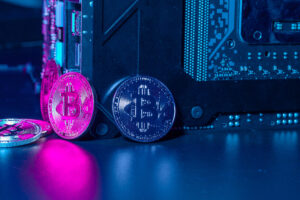
Short Address Attack in Ethereum: An In-Depth Analysis
Mechanism of Short Address Attack
A Solidity Short Address Attack exploits the padding mechanism used by Ethereum clients when an address shorter than 20 bytes is provided. In Ethereum, addresses must be 20 bytes long, but if an address of a shorter length is supplied, some clients automatically pad the address with zeros. This padding can lead to incorrect parsing of subsequent transaction data.
How the Attack Works
- Transaction Crafting: An attacker crafts a transaction with an address shorter than 20 bytes.
- Padding: The Ethereum client pads the short address with zeros to reach the required 20 bytes.
- Parameter Misalignment: This padding misaligns subsequent parameters in the transaction, causing the smart contract to interpret the transaction data incorrectly.
Example of a Vulnerable Function
Consider a smart contract with a function that transfers tokens:
function transfer(address _to, uint256 _amount) public {
// Transfer logic
}
In a ethereum short address attack, the attacker provides an address like 0x123456
instead of a full 20-byte address. When the Ethereum client pads this address with zeros, the _amount
parameter gets misaligned.
Detailed Attack Scenario
- Attack Transaction: The attacker sends a transaction to the
transfer
function with a short address0x123456
and an amount1000
. - Padding and Misalignment: The Ethereum client pads
0x123456
to0x1234560000000000000000000000000000000000
. This shifts the intended1000
amount parameter, causing the contract to interpret the data incorrectly.
Real-World Examples of Short Address Attacks
Vulnerable Token Contracts
Many ERC-20 token contracts have been vulnerable to solidity short address attacks. For example, in 2017, several token contracts were exploited using short addresses, leading to significant financial losses.
Example Code
contract VulnerableToken {
mapping(address => uint256) public balances;
function transfer(address _to, uint256 _amount) public {
require(balances[msg.sender] >= _amount, “Insufficient balance”);
balances[msg.sender] -= _amount;
balances[_to] += _amount;
}
}
In this example, an attacker could exploit the transfer
function by providing a short address and causing a misalignment in the _amount
parameter.
Preventing Short Address Attacks
Strict Input Validation
Ensure that all addresses provided as inputs are exactly 20 bytes long. This can be achieved through strict input validation.
Example of Address Length Validation
function transfer(address _to, uint256 _amount) public {
require(_to != address(0), “Invalid address”);
require(balances[msg.sender] >= _amount, “Insufficient balance”);
balances[msg.sender] -= _amount;
balances[_to] += _amount;
}
Use of ABI Encoding Functions
Use Solidity’s built-in ABI encoding functions, which ensure proper handling of input parameters and prevent misalignment.
Example of ABI Encoding
function transfer(address _to, uint256 _amount) public {
require(_to != address(0), “Invalid address”);
(bool success, bytes memory data) = address(this).call(
abi.encodeWithSelector(this.transfer.selector, _to, _amount)
);
require(success, “Transfer failed”);
}
Implementing Checksums
Ethereum addresses with checksums can reduce the risk of solidity short address attacks. Checksums ensure that addresses are correctly formatted and less likely to be tampered with.
Example of Checksums
function transfer(address _to, uint256 _amount) public {
require(_to != address(0), “Invalid address”);
require(isValidChecksumAddress(_to), “Invalid checksum address”);
balances[msg.sender] -= _amount;
balances[_to] += _amount;
}
function isValidChecksumAddress(address _addr) internal pure returns (bool) {
// Implement checksum validation logic
}
Regular Security Audits
Conduct regular security audits with professional auditors to identify and mitigate potential vulnerabilities, including those related to solidity short address attacks.
Advanced Strategies for Secure Smart Contracts
Formal Verification
Formal verification involves mathematically proving the correctness of smart contracts. This technique can be used to ensure that input parameters are handled correctly, preventing short address attacks.
Example of Formal Verification Tool
- Solidity SMTChecker: Integrated into the Solidity compiler, it uses formal methods to detect logical errors.
Static Analysis Tools
Use static analysis tools to identify vulnerabilities related to short address attacks and other security issues.
Example of Static Analysis Tool
- MythX: A security analysis tool for Ethereum smart contracts that can detect various vulnerabilities, including soliditiy short address attacks.
- Slither: A static analysis framework for Solidity.
Conclusion
Ethereum Short address attacks in Ethereum pose significant risks to the security and integrity of smart contracts. By understanding the mechanism of these attacks and implementing best practices such as strict input validation, using ABI encoding functions, implementing checksums, and conducting regular security audits, developers can mitigate these risks and enhance the security of the Ethereum ecosystem.
Source
- Survey of Security Supervision on Blockchain: This paper discusses how short address attacks utilize the EVM’s automatic completion mechanism to manipulate transaction parameters. It emphasizes the need for thorough input validation to prevent such vulnerabilities. Read more here.
- Smart Contract Best Practices by ConsenSys: This guide includes a section on short address attacks and other common vulnerabilities in smart contracts. It provides best practices for securing contracts against these and other attacks. Read more here.
- Solidity Security: Comprehensive List of Known Attack Vectors: This blog post by SigmaPrime provides a detailed list of known attack vectors, including short address attacks, and offers preventative techniques and real-world examples. Read more here.
- Enhancing Smart-Contract Security through Machine Learning: This survey explores various approaches and techniques for detecting smart contract vulnerabilities, including machine-learning methods that can identify potential short address attacks. Read more here.