Unlocking Security in Solidity with Keccak256 Hashing
Contents
#EnterTheSmartContractSecuritySeries0040
Unlocking Security in Solidity with Keccak256 Hashing
Introduction
In the world of Ethereum and Solidity, data integrity and security are paramount. The keccak256
hashing function, integral to the Ethereum protocol, plays a critical role in securing applications by creating irreversible and unique hashes. This article explores how keccak256
enhances security and maintains data integrity in Solidity smart contracts.
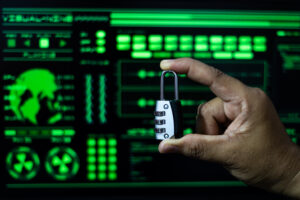
Unlocking Security in Solidity with Keccak256 Hashing
What is Keccak256?
Keccak256
is a cryptographic hashing function and a core component of the Ethereum blockchain’s security. It is used to compute unique hashes that are theoretically collision-resistant, meaning it’s highly improbable for two different inputs to produce the same output hash.
Characteristics of Keccak256
- Security: Provides strong cryptographic security, making it suitable for generating digital fingerprints of data.
- Efficiency: Highly efficient in terms of computation, making it ideal for the processing constraints within blockchain operations.
- Versatility: Used in various aspects of Ethereum, including address generation, transaction hashing, and in the implementation of smart contracts.
Using Keccak256 in Solidity
Keccak256
is utilized in Solidity through the built-in function keccak256()
, which accepts a single bytes memory argument and returns a bytes32 value representing the hash.
Syntax and Basic Usage:
function generateHash(string memory _input) public pure returns (bytes32) {
return keccak256(abi.encodePacked(_input));
}
In this example, the function generateHash
takes a string input, encodes it, and passes it to keccak256
to produce a hash. This hash can be used to verify the integrity of the data at any point without revealing the original data.
Applications of Keccak256 in Smart Contracts
Verifying Data Integrity
- Document Timestamping: Store the hash of document contents to prove existence without revealing the actual content.
- Complex Conditionals: Use hashes to validate conditions without exposing the criteria until they are met.
Generating Unique Identifiers
- Token Creation: Generate unique identifiers for digital assets or tokens based on specific attributes.
- User Anonymity: Create pseudonymous identifiers for users to interact with contracts without revealing actual identities.
Security Measures
- Commit-Reveal Schemes: Implement commit-reveal schemes in voting systems or auctions, where participants commit a hashed version of their choices or bids and reveal them later for verification.
Best Practices for Using Keccak256
Data Handling
- Consistent Data Formatting: Ensure consistent formatting of input data to avoid discrepancies in hash outputs.
- Consider Gas Costs: Be mindful of the gas costs associated with data encoding and hashing, especially with larger or more complex data structures.
Security Considerations
- Avoid Reliance on Hash Inversion: Never assume hashes can be inverted; use hashes for verification, not for encoding sensitive data.
- Update Practices as Needed: Stay updated on cryptographic best practices and potential vulnerabilities associated with hashing functions.
Conclusion
The keccak256
hashing function is a powerful tool in Solidity for maintaining data integrity, enhancing security, and facilitating complex functionalities within smart contracts. By understanding and applying keccak256
effectively, developers can greatly enhance the robustness and reliability of their blockchain applications.
Resources on Keccak256 and Solidity
Official Documentation and Guides
- Solidity Documentation: Provides detailed information on using
keccak256
within Solidity contracts.
Cryptographic Hash Functions and Security
- Ethereum GitHub Repository: The Ethereum Foundation’s official GitHub page, which includes discussions and implementations related to cryptographic functions, including
keccak256
. - Crypto101: An introductory book on cryptography that covers the basics of hash functions and their properties.
Practical Applications and Examples
- Medium Articles on Smart Contract Security: Various articles that discuss practical uses of hash functions in smart contracts and common security considerations.
- Ethereum Stack Exchange: A Q&A site where developers discuss issues and solutions related to Ethereum, including specific use cases of
keccak256
.
Advanced Cryptographic Techniques
- Coursera Cryptography Course: Offers a more in-depth exploration of cryptographic principles that underpin functions like
keccak256
. - YouTube Tutorials on Ethereum Development: These videos often cover the practical implementation of hash functions within smart contracts.
Tools and Libraries
- Remix IDE: An online tool for Solidity development that can be used to experiment with and deploy smart contracts using
keccak256
. - OpenZeppelin: A library of secure, community-vetted smart contracts that includes implementations using cryptographic functions.