Mastering Signature Verification in Solidity: Enhancing Blockchain Security
Contents
- 1 Mastering Signature Verification in Solidity: Enhancing Blockchain Security
#EnterTheSmartContractSecuritySeries0041
Mastering Signature Verification in Solidity: Enhancing Blockchain Security
Introduction
Signature verification is a crucial aspect of blockchain technology, ensuring the authenticity and integrity of transactions. In Solidity, the ability to verify digital signatures underpins many decentralized applications (dApps), from confirming ownership to executing secure and trustless transactions. This article provides a deep dive into how signature verification works in Solidity and its importance in enhancing blockchain security.
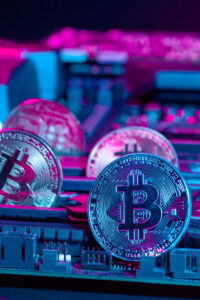
Mastering Signature Verification in Solidity: Enhancing Blockchain Security
What is Signature Verification?
Digital signature verification is the process of validating the authenticity of a message or transaction on the blockchain using cryptographic techniques. It confirms that a message or transaction was indeed sent by the claimed sender without any alteration in transit.
Key Components of Digital Signatures
- Public Key: Used to verify the signature.
- Private Key: Used by the sender to create the signature.
- Signature: Cryptographic proof that some data was approved by the possessor of the private key.
How Signature Verification Works in Solidity
Solidity uses the Ethereum Virtual Machine (EVM) capabilities to implement signature verification through a combination of built-in functions and cryptographic algorithms.
Ethereum’s ECDSA
Ethereum uses the Elliptic Curve Digital Signature Algorithm (ECDSA) for generating and verifying signatures. Solidity provides native support for ECDSA operations through the ecrecover
function, which returns the address associated with a public key that was used to sign a given piece of data.
Example: Implementing Signature Verification
// Solidity snippet demonstrating the use of ecrecover to verify a signature
contract VerifySignature {
function recoverSigner(bytes32 _hash, bytes memory _signature)
public
pure
returns (address)
{
(uint8 v, bytes32 r, bytes32 s) = splitSignature(_signature);
return ecrecover(_hash, v, r, s);
}
function splitSignature(bytes memory _sig)
internal
pure
returns (uint8, bytes32, bytes32)
{
require(_sig.length == 65, “Invalid signature length”);
bytes32 r;
bytes32 s;
uint8 v;
assembly {
r := mload(add(_sig, 32))
s := mload(add(_sig, 64))
v := byte(0, mload(add(_sig, 96)))
}
return (v, r, s);
}
}
This Solidity code demonstrates how to extract the signature components (r, s, v) and use them with ecrecover
to determine the signer’s address.
Applications of Signature Verification in Solidity
- Authentication: Verify user identities before executing transactions.
- Smart Contract Security: Ensure that interactions are authorized by signature verification.
- Non-repudiation: Prevents the sender from denying the authenticity of their signature on transactions.
Best Practices for Signature Verification
- Data Handling: Always hash data before signing to prevent exposing raw data.
- Security: Protect private keys rigorously; any exposure can lead to forgery.
- Testing and Audits: Regularly test and audit the code that handles signatures to prevent vulnerabilities.
Conclusion
Signature verification is a powerful tool in the Solidity developer’s toolkit, providing a robust method for enhancing security and trust in blockchain applications. By understanding and implementing signature verification correctly, developers can ensure that their applications are secure, authentic, and comply with the highest standards of blockchain security.
Resources on Signature Verification and Solidity
Official Documentation and Guides
- Solidity Documentation: Provides detailed information on using cryptographic functions, including
ecrecover
, within Solidity contracts. - Ethereum Foundation: Offers extensive resources on Ethereum’s cryptographic standards and practices.
Cryptographic Functions and Blockchain Security
- CryptoZombies Tutorial: An interactive coding tutorial that covers Ethereum, Solidity, and building dApps, including modules on security practices like signature verification.
- “Mastering Ethereum” by Andreas M. Antonopoulos and Gavin Wood: A comprehensive book that includes discussions on Ethereum’s cryptographic operations, including ECDSA and signature verification.
- Available for purchase or free under a creative commons license at GitHub – Mastering Ethereum
Practical Applications and Examples
- Medium Articles and Blogs: Various articles that delve into practical use cases of signature verification in Solidity and common security considerations.
- Ethereum Stack Exchange: A Q&A site where developers discuss issues and solutions related to Ethereum, including specific scenarios involving signature verification.
Online Courses and Tutorials
- Coursera and Udemy Courses: Offer courses on blockchain technology that include modules on Ethereum programming and cryptographic functions.
Development Tools and Libraries
- Remix IDE: An online IDE for Ethereum and Solidity development that can be used to test and deploy smart contracts that use signature verification.
- OpenZeppelin Library: Contains secure, community-vetted smart contracts and libraries, including those related to security and signature verification.