Streamlining Smart Contracts: Advanced Gas Saving Strategies in Solidity
Contents
#EnterTheSmartContractSecuritySeries0042
Streamlining Smart Contracts: Advanced Gas Saving Strategies in Solidity
Introduction
In the Ethereum network, every transaction costs gas, and optimizing gas usage is crucial for the efficiency and cost-effectiveness of smart contracts. This article delves into advanced strategies for minimizing gas consumption in Solidity, offering practical tips and coding practices that can help developers optimize their contracts.
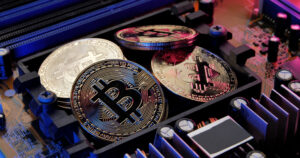
Streamlining Smart Contracts: Advanced Gas Saving Strategies in Solidity
Understanding Gas in Ethereum
Gas in Ethereum is a unit that measures the computational effort required to execute operations like transactions and smart contract functions. Efficient gas usage not only saves money but also enhances the performance and scalability of applications.
Key Factors Affecting Gas Usage:
- Complexity of Functions: More complex operations require more computational power, thus consuming more gas.
- Storage Operations: Storing data on the blockchain is expensive; efficient data handling can significantly reduce costs.
- Network Conditions: During times of network congestion, gas prices can increase dramatically.
Advanced Gas Optimization Techniques
1. Minimize On-Chain Storage
- Use Tight Variable Packing: Solidity allows multiple
uint8
orbool
types to be packed into a single storage slot if they are declared consecutively, reducing the amount of required storage. - Delete Unnecessary Storage: Using the
delete
keyword on storage variables when they are no longer needed refunds gas.
2. Optimize Function Execution
- Short-Circuiting in Conditional Statements: Order conditions in
if
statements so that the most likely false condition is checked first. - Reuse Computed Values: Store computed values in local variables if they are used multiple times within a function.
3. Efficient Use of Memory and Calldata
- Memory Instead of Storage for Temporary Variables: Use memory for intermediate variables in function calls to avoid expensive storage costs.
- Calldata Optimization: When passing arrays or complex data types to functions, use
calldata
instead ofmemory
for external functions to save gas.
4. Reduce Transaction Data
- Compress Inputs: When possible, encode multiple boolean flags or small integers into single variables to reduce the transaction data size.
- Use External Functions: Declare functions that are only called externally as
external
instead ofpublic
to save gas, asexternal
functions can read arguments directly from calldata.
5. Smart Contract Design Patterns
- Use Libraries: For reusable code, deploy libraries once and call their functions from other contracts to spread the gas cost of deployment across all users.
- Proxy Contracts for Upgradability: Use proxy patterns to separate logic from data, minimizing the need to deploy large contracts multiple times.
Practical Examples
Let’s consider a simple token contract where we apply some of these optimization techniques:
contract Token {
mapping(address => uint256) private balances;
function transfer(address _to, uint256 _amount) external {
require(balances[msg.sender] >= _amount, “Insufficient balance”);
balances[msg.sender] -= _amount;
balances[_to] += _amount;
}
}
Optimization: Use unchecked
around arithmetic operations if you are certain there will be no overflow to save gas.
Conclusion
Optimizing gas usage in Solidity is crucial for developing cost-effective and efficient smart contracts on the Ethereum network. By employing advanced optimization techniques, developers can significantly reduce the gas costs associated with deploying and interacting with smart contracts, leading to more scalable and sustainable applications.
Resources on Gas Optimization in Solidity
Official Documentation and Guides
- Solidity Documentation: Offers detailed explanations on gas costs and optimizations directly related to Solidity coding practices.
- Ethereum Gas Station: A tool and resource to understand real-time gas usage on the Ethereum network, providing insights on gas optimization.
Books and Educational Materials
- “Mastering Ethereum” by Andreas M. Antonopoulos and Gavin Wood: A thorough resource that covers Ethereum technology, including smart contract programming and gas optimization techniques.
- Available for purchase or free under a creative commons license at GitHub – Mastering Ethereum
Online Courses and Tutorials
- CryptoZombies: An interactive coding school that teaches Ethereum and Solidity programming with a focus on practical applications, including gas optimization.
- Coursera Blockchain Specialization: Covers blockchain fundamentals and Solidity programming, with modules on efficient coding practices to reduce gas costs.
Blogs and Articles
- ConsenSys Developers: Articles and guides from one of the leading Ethereum development studios, with many posts dedicated to best practices in Solidity, including gas optimization.
- OpenZeppelin Blog: Provides professional insights into smart contract development and security, including detailed discussions on optimizing gas usage.
Community and Q&A
- Ethereum Stack Exchange: A vibrant community of Ethereum enthusiasts and developers, where you can ask questions and share knowledge about gas optimization and other Solidity topics.
Tools for Optimization
- Remix IDE: An open-source web and desktop application that helps in writing, deploying, and administering Solidity smart contracts, including tools for analyzing gas usage.
- Truffle Suite: Provides development tools for Ethereum, including Truffle, which features built-in support for customizing and testing gas limits and prices.