Harnessing the Power of Bitwise Operators in Solidity Programming
Contents
#EnterTheSmartContractSecuritySeries0043
Harnessing the Power of Bitwise Operators in Solidity Programming
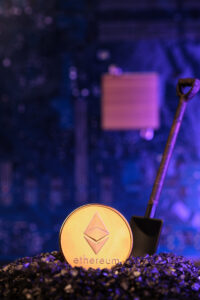
Harnessing the Power of Bitwise Operators in Solidity Programming
Introduction
Bitwise operators are fundamental tools in programming that manipulate individual bits within binary representations of numbers. In Solidity, the smart contract programming language for Ethereum, these operators play a crucial role in optimizing data storage and processing. This article explores how bitwise operators work in Solidity and how they can be effectively used to enhance smart contract functionality.
What are Bitwise Operators?
Bitwise operators perform operations on binary digits or bits of a number. Each operator manipulates the bits in a specific way, enabling direct control over data at the most granular level.
Types of Bitwise Operators in Solidity
- AND (
&
): Returns a one in each bit position for which the corresponding bits of both operands are ones. - OR (
|
): Returns a one in each bit position for which the corresponding bits of either or both operands are ones. - XOR (
^
): Returns a one in each bit position for which the corresponding bits of either but not both operands are ones. - NOT (
~
): Inverts the bits of its operand. - Shift Left (
<<
): Shifts the bits of the first operand to the left by the number of positions specified by the second operand. - Shift Right (
>>
): Shifts the bits of the first operand to the right by the number of positions specified by the second operand.
Practical Applications of Bitwise Operators in Solidity
Efficient Data Storage
- Packing Multiple Boolean Values: Use bitwise operations to store multiple boolean flags within a single
uint
variable, saving gas and storage space.
Example:
contract BitwiseStorage {
uint public flags;
function setFlag(uint position, bool value) public {
if (value)
flags |= (1 << position); // Set bit at position
else
flags &= ~(1 << position); // Clear bit at position
}
function getFlag(uint position) public view returns(bool) {
return (flags & (1 << position)) != 0;
}
}
This contract uses bitwise AND, OR, and NOT operations to manage multiple boolean states within a single uint
.
Access Control and Permissions
- Managing User Permissions: Define roles and permissions using bits, where each bit represents a specific permission level or role.
Manipulating and Validating Data
- Checksums and Error Detection: Implement simple checksums or more complex error-detecting codes using bitwise XOR operations to ensure data integrity.
Best Practices for Using Bitwise Operators
Understanding Bit Manipulation
- Know Your Data: Be aware of how data is represented in your contracts and use bitwise operators to manipulate this data efficiently.
Minimizing Gas Costs
- Use Bitwise for Compact Storage: Where appropriate, use bitwise operators to reduce the number of state variables, thus minimizing storage costs and gas usage during transactions.
Ensuring Readability
- Maintain Code Clarity: While bitwise operations can make your code more efficient, they can also reduce readability. Use comments generously to explain why and how you are using bitwise operators.
Conclusion
Bitwise operators in Solidity offer powerful ways to manipulate data directly at the bit level, providing efficiency and precision in smart contract development. By understanding and utilizing these operators appropriately, developers can optimize their contracts for better performance and lower costs, while also implementing sophisticated data handling techniques.
Resources on Bitwise Operators in Solidity
Official Documentation and Technical Guides
- Solidity Documentation: Provides the foundational aspects of Solidity, including detailed information on operators used in the language.
Educational Platforms and Courses
- CryptoZombies: An interactive learning platform where you can code Solidity through building your own crypto-collectibles game, including sections that touch on low-level operations.
- Ethereum Blockchain Developer Bootcamp With Solidity (Udemy): Offers comprehensive training in Ethereum development, including Solidity specifics and data manipulation.
Blogs and Technical Articles
- Medium – Solidity Series: Various articles from developers and enthusiasts that explore the nuances of Solidity programming, including tips on using bitwise operators.
Books
- “Mastering Ethereum: Building Smart Contracts and DApps” by Andreas M. Antonopoulos and Gavin Wood: A thorough guide covering all aspects of Ethereum, including smart contract programming with Solidity.
Tools and Libraries
- Remix IDE: A powerful open-source tool that allows you to write, deploy, and test Solidity contracts directly in your browser, including testing how bitwise operations work in practice.
- Etherscan: While primarily a block explorer, Etherscan can be used to study how existing contracts use bitwise operators by examining verified source code.
Forums and Community Discussions
- Ethereum Stack Exchange: A robust platform for asking questions and sharing insights about Ethereum and Solidity, including detailed discussions on specific programming tactics like bitwise operations.
- Solidity GitHub Discussions: Engage with the community and developers directly involved in Solidity’s development, where you can ask questions or read through past discussions on technical topics.