Ethereum Access Control Vulnerability
Access control is a critical aspect of software security, determining who can interact with or manipulate resources within a system. In the context of Ethereum, a decentralized blockchain platform, access control mechanisms are essential for securing smart contracts and ensuring that only authorized entities can perform specific actions. This blog post explores the various access control techniques used in Ethereum, their implementation, and their significance in maintaining the integrity and security of decentralized applications (DApps).
Introduction to Access Control in Ethereum
Ethereum is a decentralized platform that enables the execution of smart contracts—self-executing contracts with the terms of the agreement directly written into code. Given the immutable and public nature of the blockchain, it is crucial to implement robust access control mechanisms to protect these contracts from unauthorized access and potential malicious activities.
Importance of Access Control
Access control in Ethereum serves several vital functions:
- Security: Prevents unauthorized users from executing sensitive functions.
- Data Integrity: Ensures that only trusted entities can modify the state of the contract.
- Compliance: Helps in meeting regulatory requirements by restricting access to sensitive data.
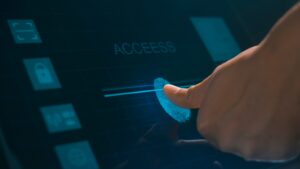
Ethereum Access Control Vulnerability
Ethereum Smart Contracts and Access Control
Smart contracts on Ethereum are written in Solidity, a high-level programming language designed for creating smart contracts. Access control in Solidity is typically managed through modifier functions, role-based access control (RBAC), and ownership patterns.
Modifier Functions
Modifiers are used in Solidity to change the behavior of functions. They are particularly useful for implementing access control.
Example of a Modifier
contract AccessControlled {
address public owner;
constructor() {
owner = msg.sender;
}
modifier onlyOwner() {
require(msg.sender == owner, “Caller is not the owner”);
_;
}
function restrictedFunction() public onlyOwner {
// Function logic
}
}
In this example, the onlyOwner
modifier restricts access to the restrictedFunction
, ensuring that only the contract owner can execute it.
Role-Based Access Control (RBAC)
RBAC is a more flexible access control model that allows assigning different roles to different addresses. This model is implemented using mappings to track roles and permissions.
Example of RBAC Implementation
contract RBAC {
mapping(address => bool) public admins;
mapping(address => bool) public users;
modifier onlyAdmin() {
require(admins[msg.sender], “Caller is not an admin”);
_;
}
modifier onlyUser() {
require(users[msg.sender], “Caller is not a user”);
_;
}
constructor() {
admins[msg.sender] = true;
}
function addAdmin(address _admin) public onlyAdmin {
admins[_admin] = true;
}
function addUser(address _user) public onlyAdmin {
users[_user] = true;
}
function restrictedAdminFunction() public onlyAdmin {
// Function logic
}
function restrictedUserFunction() public onlyUser {
// Function logic
}
}
Here, two roles—admin
and user
—are defined, with corresponding access restrictions on different functions.
Ownership Patterns
The ownership pattern is a common approach to access control in Ethereum. It typically involves a single owner who has special privileges, such as the ability to transfer ownership or grant access rights.
Ownable Contract Example
OpenZeppelin, a popular library for secure smart contract development, provides a robust Ownable
contract implementation.
pragma solidity ^0.8.0;
import “@openzeppelin/contracts/access/Ownable.sol”;
contract OwnableExample is Ownable {
function restrictedFunction() public onlyOwner {
// Function logic
}
}
By inheriting from OpenZeppelin’s Ownable
contract, developers can easily implement owner-based access control.
Advanced Access Control Techniques
Multi-Signature Wallets
A multi-signature (multi-sig) wallet requires multiple parties to sign off on a transaction before it is executed. This adds an extra layer of security, ensuring that no single entity has unilateral control over the funds.
Multi-Sig Contract Example
contract MultiSigWallet {
address[] public owners;
uint public requiredSignatures;
mapping(address => bool) public isOwner;
mapping(uint => Transaction) public transactions;
mapping(uint => mapping(address => bool)) public confirmations;
uint public transactionCount;
struct Transaction {
address destination;
uint value;
bool executed;
}
modifier onlyOwner() {
require(isOwner[msg.sender], “Caller is not an owner”);
_;
}
modifier notExecuted(uint _txId) {
require(!transactions[_txId].executed, “Transaction already executed”);
_;
}
modifier confirmed(uint _txId, address _owner) {
require(confirmations[_txId][_owner], “Transaction not confirmed by this owner”);
_;
}
constructor(address[] memory _owners, uint _requiredSignatures) {
require(_owners.length > 0, “Owners required”);
require(_requiredSignatures > 0 && _requiredSignatures <= _owners.length, “Invalid number of required signatures”);
for (uint i = 0; i < _owners.length; i++) {
address owner = _owners[i];
require(owner != address(0), “Invalid owner”);
require(!isOwner[owner], “Owner not unique”);
isOwner[owner] = true;
owners.push(owner);
}
requiredSignatures = _requiredSignatures;
}
function submitTransaction(address _destination, uint _value) public onlyOwner {
uint txId = transactionCount++;
transactions[txId] = Transaction({
destination: _destination,
value: _value,
executed: false
});
confirmations[txId][msg.sender] = true;
}
function confirmTransaction(uint _txId) public onlyOwner notExecuted(_txId) {
confirmations[_txId][msg.sender] = true;
executeTransaction(_txId);
}
function executeTransaction(uint _txId) internal notExecuted(_txId) {
if (isConfirmed(_txId)) {
Transaction storage txn = transactions[_txId];
txn.executed = true;
(bool success, ) = txn.destination.call{value: txn.value}(“”);
require(success, “Transaction execution failed”);
}
}
function isConfirmed(uint _txId) public view returns (bool) {
uint count = 0;
for (uint i = 0; i < owners.length; i++) {
if (confirmations[_txId][owners[i]]) {
count += 1;
}
if (count == requiredSignatures) {
return true;
}
}
return false;
}
}
This example demonstrates a basic multi-sig wallet where transactions require multiple confirmations before execution.
Time-Based Access Control
Time-based access control mechanisms restrict access to certain functions based on time conditions, adding an additional layer of security.
Example of Time-Based Access Control
contract TimeLocked {
uint public unlockTime;
constructor(uint _duration) {
unlockTime = block.timestamp + _duration;
}
modifier onlyAfterUnlock() {
require(block.timestamp >= unlockTime, “Function is locked”);
_;
}
function lockedFunction() public onlyAfterUnlock {
// Function logic
}
}
In this example, the lockedFunction
can only be called after a specified duration has passed since the contract deployment.
Implications and Best Practices
Security Implications
Implementing access control correctly is paramount to the security of Ethereum smart contracts. Inadequate access control can lead to vulnerabilities such as unauthorized access, data breaches, and financial losses.
Best Practices
- Use Proven Libraries: Utilize well-tested libraries like OpenZeppelin to implement access control mechanisms.
- Follow the Principle of Least Privilege: Grant the minimum necessary permissions to users and roles.
- Regular Audits: Conduct regular security audits to identify and rectify potential vulnerabilities.
- Implement Multi-Sig Wallets: Use multi-signature wallets for managing significant assets to reduce the risk of unauthorized transactions.
- Time-Based Restrictions: Apply time-based access control for critical functions to mitigate the risk of immediate exploitation.
Conclusion
Access control is a fundamental aspect of Ethereum smart contract security. By leveraging techniques such as modifier functions, role-based access control, ownership patterns, multi-signature wallets, and time-based restrictions, developers can create secure and robust smart contracts. Understanding and implementing these mechanisms is essential for protecting decentralized applications from unauthorized access and ensuring their reliable operation in the blockchain ecosystem.
Source
- The State of Ethereum Smart Contracts Security: This paper reviews the latest advancements and research on Ethereum smart contract security, identifying 13 vulnerabilities and their countermeasures. It discusses various security-analysis tools and highlights inconsistencies in vulnerability definitions, which complicate identification and categorization. This source can provide a comprehensive overview of common vulnerabilities and the tools available for detection and mitigation (MDPI).
- Ethereum Smart Contract Vulnerability Detection Model: This study proposes a novel detection scheme combining metric learning and a bidirectional long short-term memory (BiLSTM) network to optimize the feature space for detecting vulnerabilities. It evaluates four types of vulnerabilities, including inconsistent access controls, achieving high detection accuracy rates. This source is useful for understanding advanced detection methodologies and their effectiveness (MDPI).
- Survey of Smart Contract Security Analysis Methods: This survey covers various methods for analyzing smart contract security, including static and dynamic analysis, formal verification, and runtime monitoring. It provides insights into the strengths and limitations of different approaches, making it a valuable reference for understanding the current landscape of security analysis techniques (ar5iv).