Introduction Smart Contract ‘Hello World
Contents
#EnterTheSmartContractSecuritySeries001
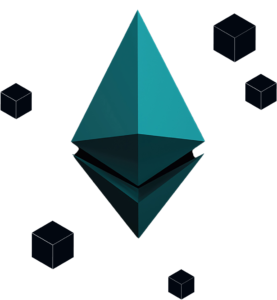
Welcome to the first post in our exciting #EnterTheSmartContractSecurity series!
In this series, we will delve deeper into the world of the Solidity language and learn about its fascinating features, and we will begin to explore the power of Solidity through the “Hello World” tradition.
#EnterTheSmartContractSecuritySeries001 “Hello World”
Get ready to embark on an educational adventure full of technical insights, engaging content and even a touch of technical brilliance! Let’s roll up our sleeves to unlock the potential of Solidity and learn how to create our own “Hello World” contract.
Let’s Simplify Smart Contracts!
The “Hello World” Tradition:
At first glance, “Hello World” may seem like a simple and overused example, but it is a critical starting point when learning any programming language. In Solidity, it provides a foundation for more complex smart contracts while allowing us to grasp basic concepts and syntax. Let’s begin our exploration by examining the code structure and understanding its components.
Code Structure:
Pragma Directive:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.17;
contract HelloWorld {
// Contract codes start here
}
The starting point of every Solidity contract is the pragma directive. It specifies the compiler version to use. In our example, we set it to require version 0.8.17 or higher and be compatible with a version lower than 0.9.0. This ensures compatibility and maintains stability while taking advantage of the latest improvements.
Contract Notification:
Next, we declare our contract with the keyword “contract”, in this example the contract name is “HelloWorld.” Contracts are the building blocks of smart contracts, encapsulating functionality and data as reusable units.
State Variable:
string public greet = “Hello World!”;
In a contract, we define a state variable of type “string” called “greet”. State variables store data for the lifetime of the contract. The “public” keyword makes the variable externally accessible, so we can read the value.
Initialization:
In our example, we initialize the variable “greet” with the string “Hello World!” by setting the initial greeting message. This sets the initial greeting message and can be changed later.
Functions: Read and Update Data:
function greetMe() public view returns (string memory) {
return greet;
}
function setGreet(string memory _greet) public {
greet = _greet;
}
Description: greetMe() returns the current greeting. setGreet() is used to set a new greeting. This increases the interactivity of the contract.
Access Control and Security:
address owner;
constructor() {
owner = msg.sender;
}
modifier onlyOwner() {
require(msg.sender == owner, “Only the contract owner can call this function.”);
_;
}
function setGreetAsOwner(string memory _greet) public onlyOwner {
greet = _greet;
}
Description:
The owner variable holds the address of the contract owner. the constructor() function runs when the contract is uploaded to the blockchain and sets the address of the contract uploader to owner. the onlyOwner modifier allows only the contract owner to perform some operations. the setGreetAsOwner() function allows only the contract owner to update the greeting using this control.
Smart Contract Technical Overview:
After reviewing the code structure, let’s dive deeper into the technical aspects of Solidity.
Solidity Version Update:
Solidity is a rapidly evolving language and it is vital to ensure compatibility with different compiler versions. By specifying with the pragma directive, we ensure that our contract is compiled using a specific Solidity compiler version. This avoids potential problems due to changes in new compiler versions.
Smart Contract Data Types:
Solidity offers various data types to meet different needs. In our example, we used the “string” data type to store the greeting message. Solidity also supports other primitive types such as integers, booleans, addresses and more. Understanding these data types is essential for building robust and efficient smart contracts.
Visibility Tokens:
By using a “public” visibility token, we open the state variable “greet” to the outside world. Solidity provides various visibility tokens such as public, private, internal and external that control the accessibility of variables and functions. Defining visibility correctly is vital for security and encapsulation.
Smart Contract Deployment and Interaction:
Once we have written our Solidity contract, we need to deploy it to a blockchain network to allow users to interact with functions and access their data. Contract deployment involves compiling the Solidity code, creating a bytecode representation, and deploying it to the desired blockchain network using tools like Remix or Truffle.
Default Access and Visibility:
In Solidity, four types of visibility access are available for functions and variables: public, private, external and internal. The use of each type of access can have a major impact on the security and efficiency of the contract.
Public:
Accessible both externally and from inside the contract. Generally used for data read operations.
Private:
Accessible only within the contract where it is defined. Ideal for particularly sensitive functions.
External:
Can only be called from outside. Generally preferred for interfaces between interfaces.
Internal:
Accessible from within the contract or from a sub-contract. Useful in inherited contracts.
Choosing the wrong access type can lead to functions being used in unexpected ways, which poses potential security risks. For example, mistakenly marking a function as public when it should only be accessed internally can lead to unintended access. It is important to note that in Solidity, the code written can be seen by anyone on the blockchain, even if it is marked as private. This requires a balanced approach between transparency and security.
Educational Content:
Solidity is a powerful language that makes it possible to build decentralized applications and smart contracts. By understanding the code structure and technical nuances, you become better equipped to build complex and secure applications on various blockchain platforms.
References
- Ethereum Foundation. (n.d.). Solidity Documentation. Retrieved from https://docs.soliditylang.org/en/v0.8.0/
- ConsenSys. (2021). Introduction to Smart Contracts. Retrieved from https://consensys.net/knowledge-base/ethereum-101/what-is-a-smart-contract/
- Remix Project. (n.d.). Remix – Ethereum IDE. Retrieved from https://remix.ethereum.org/
- Truffle Suite. (n.d.). Truffle Suite: Smart Contract Development. Retrieved from https://www.trufflesuite.com/
- Nomic Foundation. (n.d.). Hardhat: Ethereum Development Environment. Retrieved from https://hardhat.org/