Smart Contract Solidity Crafting Secure and Efficient Blockchain Solutions
Contents
#EnterTheSmartContractSecuritySeries006
Understanding Immutability in Solidity Of Crafting Trustworthy Smart Contracts
Abstract:
In the digital age, where blockchain technology is revolutionizing the way we conceptualize and implement security, transparency, and trust, the attribute of immutability in smart contracts is of paramount importance. This scholarly article embarks on an in-depth exploration of the immutable properties facilitated by Solidity, the leading programming language for Ethereum-based smart contracts. By implementing immutable variables, developers can ensure that key data elements remain unchanged once a contract is deployed, thereby securing the digital agreements against unauthorized modifications and breaches.
Immutability, in the context of Solidity, provides a strategic advantage by ensuring that essential data persists unchanged throughout the lifecycle of a contract, fostering an environment of trust and reliability. This paper investigates how immutability not only secures smart contracts but also optimizes their execution and efficiency. Through a rigorous examination of theoretical concepts backed by practical implementations, this article aims to illuminate the critical role immutability plays in building reliable, secure, and efficient smart contracts, thereby enhancing the overall robustness of blockchain applications.
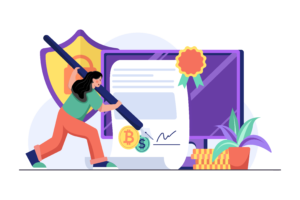
Solidity Smart Contract Crafting Secure and Efficient Blockchain Solutions
Introduction:
The concept of immutability in programming is not merely a feature—it is a cornerstone of modern blockchain technology that ensures security, consistency, and trustworthiness. As the foundational platform for decentralized applications, the Ethereum blockchain leverages immutability primarily through the use of smart contracts, with Solidity at the forefront as its preferred programming language. This introduction explores the principle of immutability in Solidity, emphasizing its critical role in the development and deployment of secure and reliable smart contracts.
Immutability refers to the property of an object to remain unchanged over time. In the context of Solidity and smart contracts, it means that once a variable or a piece of data is written to the blockchain, it cannot be altered or deleted. This characteristic is vital for ensuring that once deployed, smart contracts operate exactly as intended without interference, making them trustless and verifiable systems crucial in scenarios ranging from financial transactions to automated governance.
Solidity introduces immutable variables as a robust feature that enhances security by preventing the alteration of key data after deployment. These variables, once set during contract initialization, cannot be modified, thus guaranteeing the integrity of the contract throughout its lifecycle. The immutable property in Solidity is crucial for maintaining the original state and behavior of deployed contracts, thus providing assurances not just to contract developers but also to users and stakeholders about the unchangeable nature of critical contractual agreements and terms.
This article will delve into how immutability is implemented in Solidity, the advantages it brings to smart contract development, and the broader implications for trust and security in blockchain applications. By examining both theoretical aspects and practical examples, this section sets the stage for a comprehensive understanding of why immutability is not just beneficial but essential in the creation of decentralized applications on Ethereum.
Chapter 1: Conceptualizing Immutability in Solidity
Defining Immutability:
In Solidity, immutability is enforced through immutable variables that are set at the time of contract creation and remain constant throughout the contract’s life. Unlike constants, immutable variables can be assigned during the construction phase, which offers flexibility combined with security.
Illustrative Example:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.17;
contract SecureStore {
address public immutable creator;
uint public immutable deploymentTime;
constructor() {
creator = msg.sender; // Set at deployment
deploymentTime = block.timestamp; // Capture the deployment time
}
}
This example showcases a contract SecureStore where the creator and deploymentTime are set immutably in the constructor. Such practices ensure that these foundational values, once set, are preserved unchanged, securing the contract against alterations.
Chapter 2: The Merits of Immutability
Enhancing Security:
Immutability restricts the post-deployment modification of critical variables, safeguarding smart contracts against tampering and ensuring operational consistency.
Reducing Costs and Complexity:
By reducing the need for getters and setters for certain variables, immutable structures simplify contract interfaces and decrease gas costs associated with state modifications.
Fostering Trust and Predictability:
Immutable variables establish a predictable environment where contract behavior can be assured, significantly important in financial and transactional applications.
Chapter 3: Implementing Immutability
Practical Applications:
Utilizing immutability in contracts like SecureStore enhances their reliability for applications requiring fixed attributes, such as registries or identity verifications.
Advanced Implementation:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.17;
contract TransactionLock {
uint public immutable lockPeriod;
constructor(uint _lockPeriod) {
lockPeriod = now + _lockPeriod;
}
function isLocked() public view returns (bool) {
return now < lockPeriod;
}
}
In TransactionLock, the lockPeriod demonstrates the use of an immutable variable to enforce a time-based condition, highlighting how immutability can govern contract functionalities securely.
Conclusion:
The strategic application of immutability in Solidity empowers developers to build smart contracts that are not only secure and cost-effective but also transparent and reliable. Embracing this feature can significantly enhance the trustworthiness and efficiency of decentralized applications on the Ethereum blockchain.
References
- Ethereum Foundation. (n.d.). Solidity Documentation. Retrieved from https://docs.soliditylang.org/en/v0.8.0/
- ConsenSys. (2021). Smart Contract Best Practices. Retrieved from https://consensys.github.io/smart-contract-best-practices/
- OpenZeppelin. (n.d.). OpenZeppelin Contracts. Retrieved from https://docs.openzeppelin.com/contracts/4.x/
- Trail of Bits. (2019). Smart Contract Security Guidance. Retrieved from https://blog.trailofbits.com/2019/07/09/smart-contract-security-guidance/
- Nomic Foundation. (n.d.). Hardhat: Ethereum Development Environment. Retrieved from https://hardhat.org/
- PeckShield. (2020). The Comprehensive Ethereum Smart Contract Security Best Practices. Retrieved from https://peckshield.medium.com/the-comprehensive-ethereum-smart-contract-security-best-practices-8c173f4eeb0e