Ethereum Unchecked Return Values For Low Level Calls Vulnerability
Unchecked return values for low-level calls in Ethereum smart contracts can introduce significant vulnerabilities and security risks. This comprehensive blog post delves into the nature of this issue, its implications, and best practices for secure smart contract development, providing a detailed examination suitable for a doctoral thesis.
Introduction to Low-Level Calls in Ethereum
Ethereum smart contracts, written in Solidity, often use low-level calls to interact with other contracts or transfer Ether. These calls include functions like call
, delegatecall
, staticcall
, and send
. While these functions offer flexibility, they also require careful handling to avoid security pitfalls.
Importance of Low-Level Calls
Low-level calls are used for various purposes in Ethereum smart contracts, such as:
- Interacting with unknown contracts.
- Forwarding calls with dynamic parameters.
- Implementing proxy patterns.
- Transferring Ether between contracts.
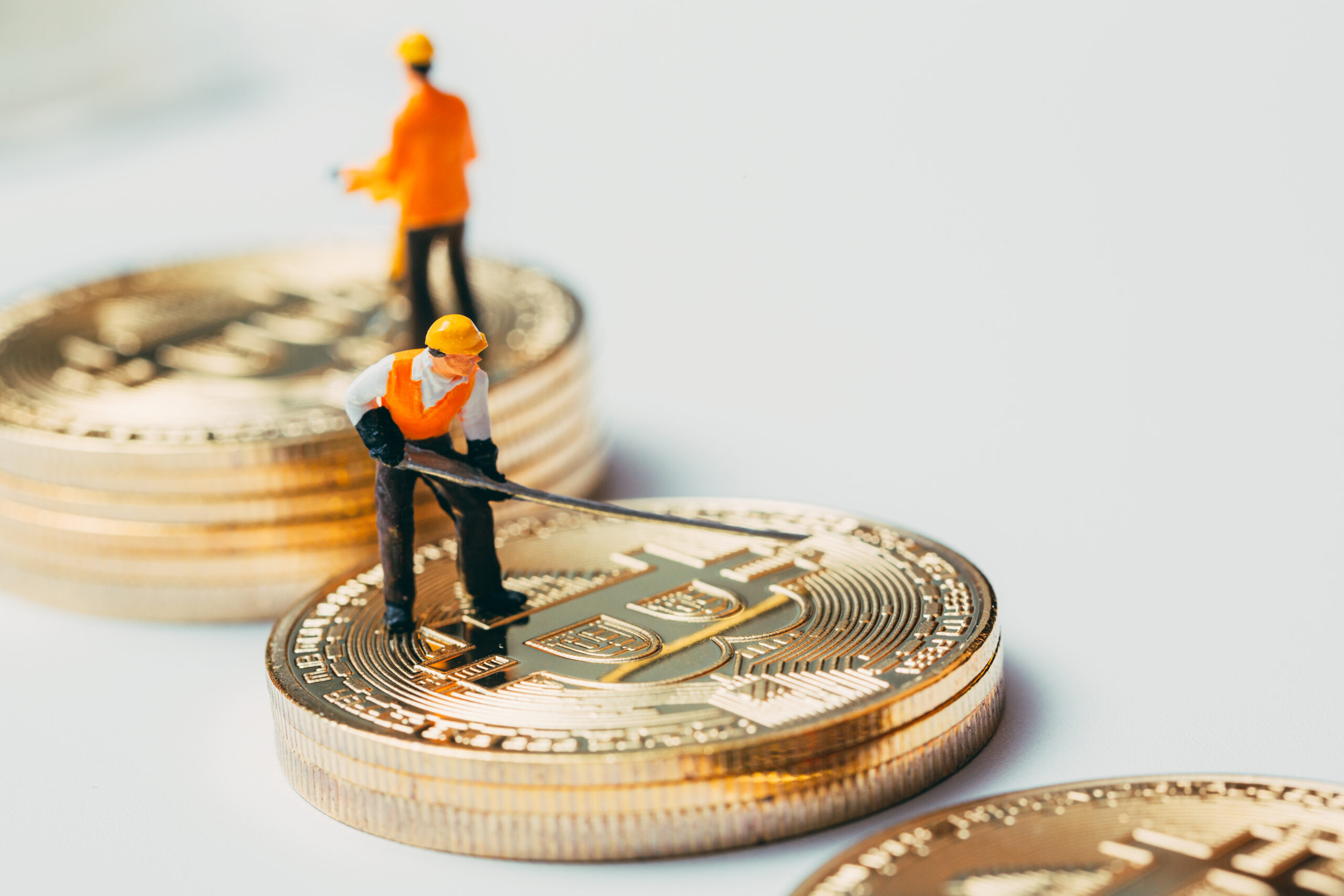
Unchecked Return Values for Low-Level Calls in Ethereum: An In-Depth Analysis
The Issue of Unchecked Return Values
One of the critical security issues in Ethereum smart contracts is the failure to check the return values of low-level calls. When a low-level call is made, it returns a boolean indicating success or failure. If this return value is not checked, the contract may continue execution under the false assumption that the call was successful, leading to potential vulnerabilities.
Understanding Unchecked Return Values
When a low-level call is made, it returns two values:
- A boolean indicating the success (
true
) or failure (false
) of the call. - A byte array containing any returned data.
Example of an Unchecked Low-Level Call
address recipient = 0x1234567890123456789012345678901234567890;
(bool success, ) = recipient.call{value: 1 ether}(“”);
// No check on `success`
In this example, if the call to transfer 1 Ether fails, the contract does not handle the failure, potentially leading to incorrect state assumptions.
Implications of Unchecked Return Values
Unchecked return values can result in various issues, including:
- Reentrancy Attacks: Attackers can exploit unchecked calls to repeatedly invoke a contract.
- Loss of Funds: Ether or tokens may be unintentionally lost if transfers fail.
- Incorrect Contract Logic: The contract may proceed with incorrect assumptions, leading to unintended behaviors.
Real-World Example: The DAO Attack
One of the most infamous incidents involving unchecked return values was the DAO (Decentralized Autonomous Organization) attack in 2016. The attacker exploited a reentrancy vulnerability, which was partly due to unchecked return values.
How the DAO Attack Occurred
The DAO contract allowed users to withdraw their funds via a low-level call. However, the return value of the call was not checked, enabling the attacker to recursively call the withdrawal function before the contract’s state was updated.
Vulnerable DAO Code
function withdraw(uint _amount) public {
if (balances[msg.sender] >= _amount) {
msg.sender.call.value(_amount)(“”);
balances[msg.sender] -= _amount;
}
}
In this code, the call to msg.sender
did not check the return value, allowing the reentrancy attack to drain funds from the contract.
Best Practices for Handling Low-Level Calls
Always Check Return Values
Ensure that the return values of low-level calls are checked and handled appropriately.
Safe Handling Example
(address recipient, uint amount) = (0x1234567890123456789012345678901234567890, 1 ether);
(bool success, ) = recipient.call{value: amount}(“”);
require(success, “Call failed”);
In this example, the require
statement ensures that the contract reverts if the call fails.
Use High-Level Abstractions
Whenever possible, use high-level functions provided by Solidity and libraries like OpenZeppelin, which handle return values and edge cases internally.
Example Using OpenZeppelin’s Safe Transfer
import “@openzeppelin/contracts/token/ERC20/utils/SafeERC20.sol”;
using SafeERC20 for IERC20;
IERC20(tokenAddress).safeTransfer(recipient, amount);
Implement Reentrancy Guards
To prevent reentrancy attacks, use reentrancy guards such as the nonReentrant
modifier provided by OpenZeppelin.
Reentrancy Guard Example
import “@openzeppelin/contracts/security/ReentrancyGuard.sol”;
contract SecureContract is ReentrancyGuard {
function withdraw(uint _amount) public nonReentrant {
require(balances[msg.sender] >= _amount, “Insufficient balance”);
(bool success, ) = msg.sender.call{value: _amount}(“”);
require(success, “Transfer failed”);
balances[msg.sender] -= _amount;
}
}
Fallback Functions and Receive Functions
Handle fallback and receive functions carefully to avoid vulnerabilities related to low-level calls.
Example of a Safe Fallback Function
fallback() external payable {
revert(“Fallback function called”);
}
Using Try/Catch for External Calls
Solidity 0.6.0 introduced the try/catch
syntax, which provides a structured way to handle errors in external calls.
Example of Try/Catch
try externalContract.someFunction() returns (uint result) {
// Handle success
} catch {
// Handle failure
revert(“External call failed”);
}
Advanced Strategies for Secure Low-Level Calls
Static Analysis Tools
Use static analysis tools to identify unchecked return values and other potential vulnerabilities in your smart contract code.
Example Tools
- MythX: A security analysis tool for Ethereum smart contracts.
- Slither: A static analysis framework for Solidity.
Formal Verification
Formal verification can mathematically prove the correctness of smart contract logic, including handling of return values.
Example of Formal Verification
- Solidity SMTChecker: Integrated into the Solidity compiler, it uses formal methods to detect logical errors.
Regular Audits
Conduct regular security audits with professional auditors to ensure that all low-level calls are properly checked and handled.
Conclusion
Unchecked return values for low-level calls in Ethereum smart contracts pose significant security risks, including reentrancy attacks, loss of funds, and incorrect contract logic. By understanding the nature of these issues and implementing best practices such as always checking return values, using high-level abstractions, implementing reentrancy guards, and employing advanced strategies like static analysis and formal verification, developers can create secure and robust smart contracts.
Source
- Solidity Security: Comprehensive list of known attack vectors and common anti-patterns: This resource provides an in-depth look at various security issues, including unchecked low-level calls, and offers recommendations for secure smart contract development. Read more here.
- Low-Level Solidity Calls In-Depth: This guide discusses the differences between
send()
,transfer()
, andcall()
, highlighting the importance of checking return values to prevent vulnerabilities. Read more here. - External Calls – Ethereum Smart Contract Best Practices by ConsenSys: This document provides best practices for handling external calls in smart contracts, emphasizing the risks of unchecked low-level calls and offering strategies to mitigate them. Read more here.
- Unchecked Low-Level Calls | Conflux Docs: This article provides examples of vulnerable contracts and explains how unchecked low-level calls can lead to security issues, along with prevention measures. Read more here.